PHP validation class: Email, password, and field validation
PHP OOP: Exercise-19 with Solution
Write a class called 'Validation' with static methods to validate email addresses, passwords, and other common input fields.
Sample Solution:
PHP Code :
<?php
class Validation {
public static function validateEmail($email) {
// Check if the email is valid using a regular expression
return filter_var($email, FILTER_VALIDATE_EMAIL) !== false;
}
public static function validatePassword($password) {
// Here's an example that checks if the password is at least 8 characters long
return strlen($password) >= 8;
}
public static function validateField($field) {
// Here's an example that checks if the field is not empty
return !empty($field);
}
}
$email = "[email protected]";
$password = "password123";
$field = "";
if (Validation::validateEmail($email)) {
echo "Email is valid.</br>";
} else {
echo "Email is invalid.</br>";
}
if (Validation::validatePassword($password)) {
echo "Password is valid.</br>";
} else {
echo "Password is invalid.v";
}
if (Validation::validateField($field)) {
echo "Field is valid.</br>";
} else {
echo "Field is invalid.</br>";
}
?>
Sample Output:
Email is valid. Password is valid. Field is invalid.
Explanation:
In the above exercise -
- The "Validation" class has three static methods: validateEmail(), validatePassword(), and validateField(), which validate email addresses, passwords, and other common input fields, respectively.
- The "validateEmail($email)" method uses the FILTER_VALIDATE_EMAIL filter to check if the email is valid according to the provided regular expression. It returns true if the email is valid, and false otherwise.
- The "validatePassword($password)" method checks if the password meets your specified requirements. In this example, it checks if the password is at least 8 characters long. It returns true if the password is valid, and false otherwise.
- The "validateField($field)" method validates other common input fields. In this example, it checks if the field is not empty. It returns true if the field is valid, and false otherwise.
Flowchart:
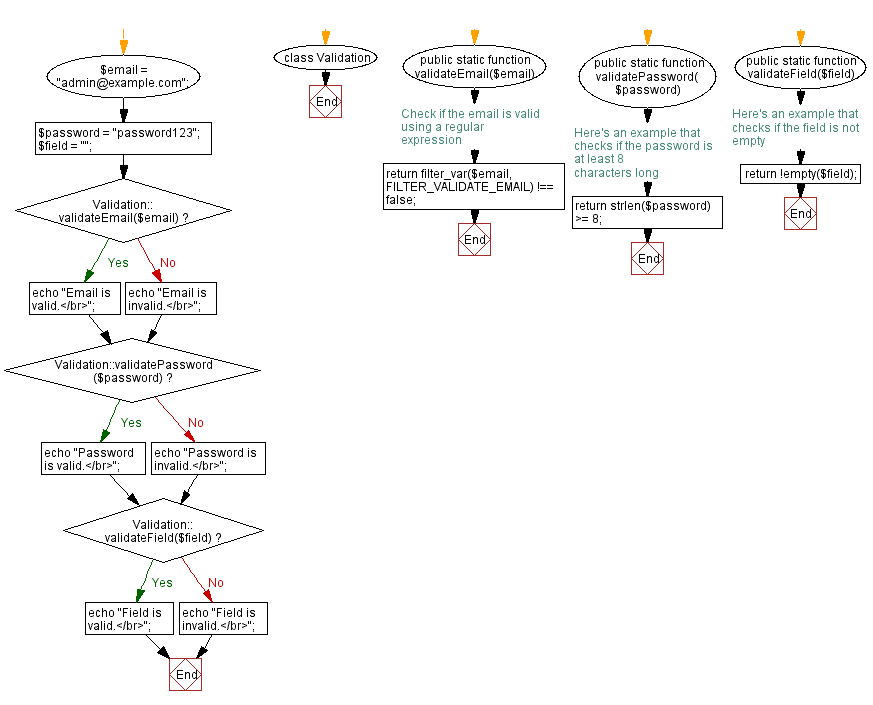
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Singleton design pattern implementation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics