PHP JSON Exercises : Display JSON decode errors
Write a PHP function to display JSON decode errors.
Sample Solution:
PHP Code:
<?php
// Define a function to handle JSON error messages
function json_error_message($json_str)
{
// Create an array to hold the JSON string
$json[] = $json_str;
// Output the JSON string
echo $json;
// Iterate through the array of JSON strings
foreach ($json as $string)
{
// Output the decoding attempt
echo 'Decoding: ' . $string."\n";
// Attempt to decode the JSON string
json_decode($string);
// Check for JSON decoding errors and output corresponding messages
switch (json_last_error())
{
case JSON_ERROR_NONE:
echo ' - No errors'."\n";
break;
case JSON_ERROR_DEPTH:
echo ' - Maximum stack depth exceeded'."\n";
break;
case JSON_ERROR_STATE_MISMATCH:
echo ' - Underflow or the modes mismatch'."\n";
break;
case JSON_ERROR_CTRL_CHAR:
echo ' - Unexpected control character found'."\n";
break;
case JSON_ERROR_SYNTAX:
echo ' - Syntax error, malformed JSON'."\n";
break;
case JSON_ERROR_UTF8:
echo ' - Malformed UTF-8 characters, possibly incorrectly encoded'."\n";
break;
default:
echo ' - Unknown error'."\n";
break;
}
}
}
// Call the function with a sample JSON string
json_error_message('{"color1": "Red Color"}');
?>
Output:
PHP Notice: Array to string conversion in /home/students/98837c40-6 86c-11e7-a315-21e19fc8c51e.php on line 5 ArrayDecoding: {"color1": "Red Color"} - No errors
Explanation:
In the exercise above,
- function json_error_message($json_str): Defines a function called "json_error_message()" that takes a JSON string as input.
- $json[] = $json_str;: Creates an array containing the provided JSON string.
- echo $json;: Outputs the JSON string.
- foreach ($json as $string) { ... }: Iterates through each JSON string in the array.
- echo 'Decoding: ' . $string."\n";: Outputs a message indicating the JSON decoding attempt.
- json_decode($string);: Attempts to decode the JSON string.
- switch (json_last_error()) { ... }: Checks for JSON decoding errors and outputs corresponding messages based on the error code.
Flowchart :
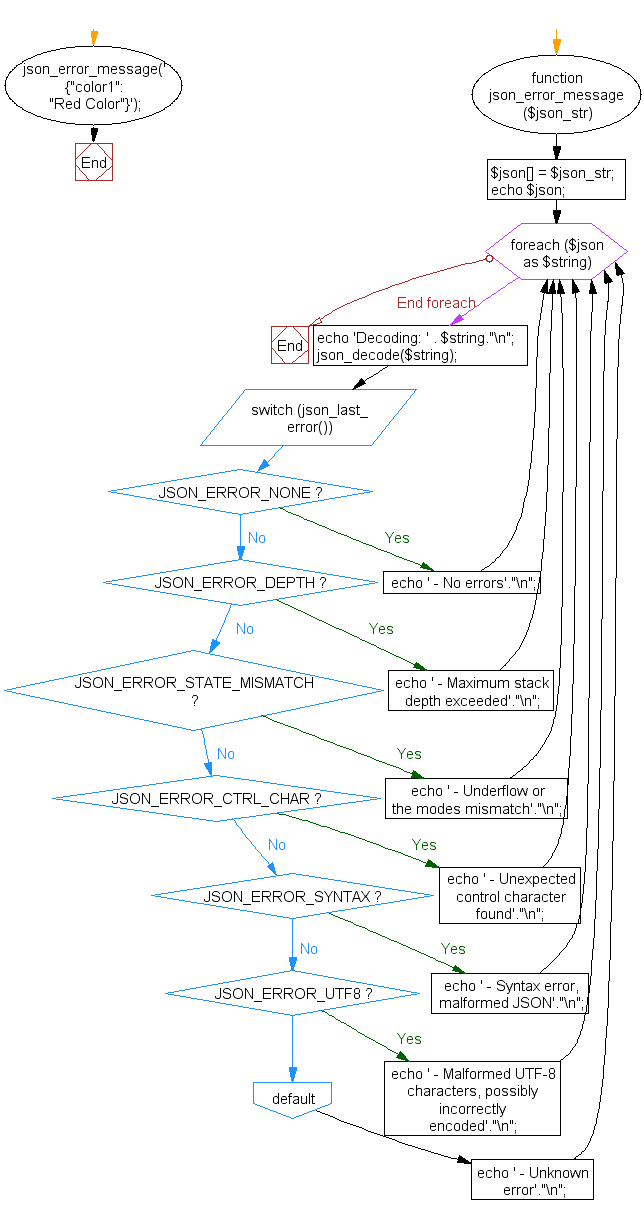
Go to:
PREV : Write a PHP script to get JSON representation of a value from an array.
NEXT : PHP Searching and Sorting Algorithm Exercises Home.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.