Delaying Print Output with asyncio Coroutines in Python
Python Asynchronous: Exercise-1 with Solution
Write a Python program that creates an asynchronous function to print "Python Exercises!" with a two second delay.
Sample Solution:
Code:
import asyncio
async def print_delayed_message():
await asyncio.sleep(2) # Wait for 2 seconds
print("Python Exercises!")
# Create an event loop and run the asynchronous function
async def main():
await print_delayed_message()
# Run the event loop
asyncio.run(main())
Output:
Python Exercises!
Explanation:
In the above exercise, the "delayed_message()" function is defined as an asynchronous coroutine. It uses the await asyncio.sleep(2) statement to pause the execution of the coroutine for 2 seconds. Then, "Python Exercises!" is printed to the console.
The main coroutine runs the delayed_message coroutine. The asyncio.run(main()) line starts the event loop, which executes the asynchronous operations defined in the coroutines.
Flowchart:
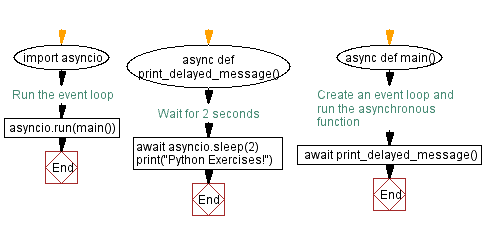
Previous: Python Asynchronous Exercises Home.
Next: Running asynchronous Python functions with different time delays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics