Python: Compare two unordered lists
36. Compare Two Unordered Lists (Not Sets)
Write a Python program to compare two unordered lists (not sets).
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Define a function 'compare_lists' that takes two lists 'x' and 'y' as arguments
def compare_lists(x, y):
# Compare whether the counts of elements in 'x' and 'y' are equal using Counter
return Counter(x) == Counter(y)
# Create two lists 'n1' and 'n2' containing integer values
n1 = [20, 10, 30, 10, 20, 30]
n2 = [30, 20, 10, 30, 20, 50]
# Call the 'compare_lists' function with 'n1' and 'n2' as arguments and print the result
print(compare_lists(n1, n2))
Sample Output:
False
Flowchart:
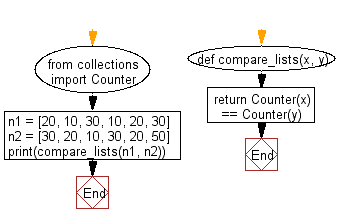
For more Practice: Solve these Related Problems:
- Write a Python program to compare two lists element-wise and return True if they contain the same elements with the same frequencies, regardless of order.
- Write a Python program to use collections.Counter to determine if two lists are equivalent without considering order.
- Write a Python program to implement a function that checks if two lists are equal by sorting them and comparing the sorted versions.
- Write a Python program to compare two lists using a dictionary to count occurrences and then determine if they are identical in content.
Go to:
Previous: Write a Python program to group a sequence of key-value pairs into a dictionary of lists.
Next: Python : Array - Exercises, Practice, Solution.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.