Python: Determine whether a given year is a leap year
Python datetime: Exercise-2 with Solution
Write a Python program to determine whether a given year is a leap year.
Sample Solution:
Python Code:
# Define a function named leap_year that takes a year (y) as input
def leap_year(y):
# Check if the year is divisible by 400
if y % 400 == 0:
return True
# Check if the year is divisible by 100 but not divisible by 400
if y % 100 == 0:
return False
# Check if the year is divisible by 4 but not divisible by 100
if y % 4 == 0:
return True
# If none of the above conditions are met, the year is not a leap year
else:
return False
# Print whether the year 1900 is a leap year or not using the leap_year function
print(leap_year(1900))
# Print whether the year 2004 is a leap year or not using the leap_year function
print(leap_year(2004))
Output:
False True
Explanation:
In the exercise above,
- The above code defines a function named "leap_year()" that takes a year (denoted by 'y') as input.
- Leap Year logic:
- Inside the function, it checks if the year is divisible by 400. If it is, it returns 'True', indicating it's a leap year because leap years are evenly divisible by 400.
- If the year is not divisible by 400, it checks if it's divisible by 100. If it is, it returns 'False' because years divisible by 100 but not by 400 are not leap years.
- If the year is not divisible by 100, it checks if it's divisible by 4. If it is, it returns 'True', indicating it's a leap year because years divisible by 4 are leap years unless they are also divisible by 100 (as handled in the previous condition).
- If none of the above conditions are met, it returns 'False', indicating it's not a leap year.
- Finally "leap_year()" functiont tests two different years (1900 and 2004) and print the results.
Flowchart:
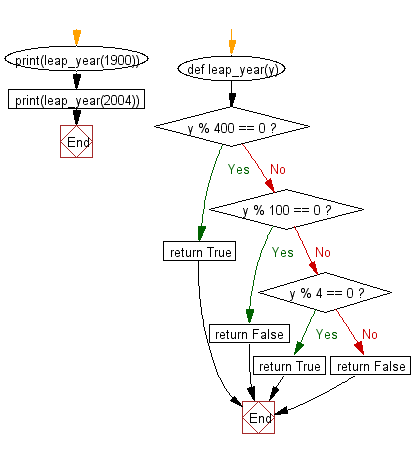
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python script to display the various Date Time formats.
Next: Write a Python program to convert a string to datetime.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics