Implementing a Python decorator for caching with expiration time in functions
Python Decorator: Exercise-12 with Solution
Write a Python program that implements a decorator to provide caching with expiration time for a function.
Sample Solution:
Python Code:
import time
def cache_with_expiry(expiry_time):
def decorator(func):
cache = {}
def wrapper(*args, **kwargs):
key = (*args, *kwargs.items())
if key in cache:
value, timestamp = cache[key]
if time.time() - timestamp < expiry_time:
print("Retrieving result from cache...")
return value
result = func(*args, **kwargs)
cache[key] = (result, time.time())
return result
return wrapper
return decorator
# Example usage
@cache_with_expiry(expiry_time=5) # Cache expiry time set to 5 seconds
def calculate_multiply(x, y):
print("Calculating product of two numbers...")
return x * y
# Call the decorated function multiple times
print(calculate_multiply(23, 5)) # Calculation is performed
print(calculate_multiply(23, 5)) # Result is retrieved from cache
time.sleep(5)
print(calculate_multiply(23, 5)) # Calculation is performed (cache expired)
Sample Output:
Calculating product of two numbers... 115 Retrieving result from cache... 115 Calculating product of two numbers... 115
Explanation:
In the above exercise,
- The "cache_with_expiry decorator" takes "expiry_time" as an argument, which determines the time in seconds after which the cached result expires.
- Inside the decorator, a cache dictionary is created to store the cached results along with their timestamps.
- The wrapper function checks if the result for a given set of arguments exists in the cache. If it does, it compares the timestamp with the current time. If the difference is within expiry_time, the cached value is returned.
- If the result is not found in the cache or if the cached result has expired, the original function is called. The result is stored in the cache along with the current timestamp.
- Finally, the wrapper function returns from the decorator.
- The decorated function, calculate_multiply, is called multiple times to demonstrate caching behavior. The first call performs the calculation, subsequent calls within the expiry time retrieve the result from the cache, and after the expiry time, a new calculation is performed.
Flowchart:
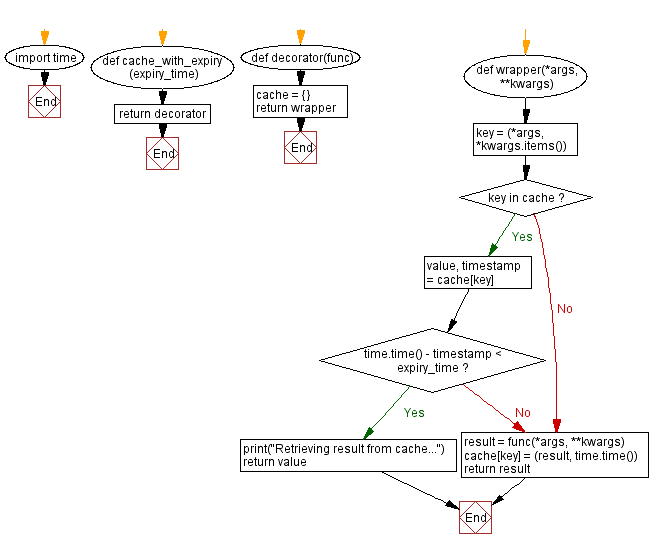
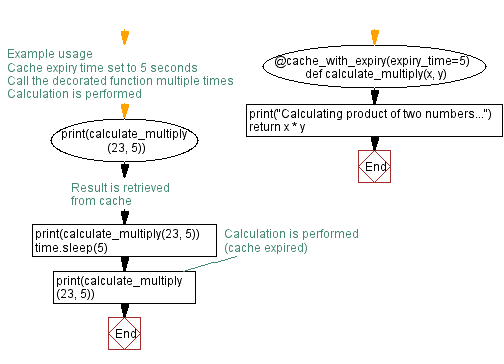
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Implementing a Python decorator to measure memory usage of a function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics