Implementing a Python decorator for function retry
Python Decorator: Exercise-6 with Solution
Write a Python program that implements a decorator to retry a function multiple times in case of failure.
Sample Solution:
Python Code:
import sqlite3
import time
def retry_on_failure(max_retries, delay=1):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(max_retries):
try:
result = func(*args, **kwargs)
return result
except Exception as e:
print(f"Error occurred: {e}. Retrying...")
time.sleep(delay)
raise Exception("Maximum retries exceeded. Function failed.")
return wrapper
return decorator
@retry_on_failure(max_retries=3, delay=2)
def connect_to_database():
conn = sqlite3.connect("example.db")
cursor = conn.cursor()
cursor.execute("SELECT * FROM users")
result = cursor.fetchall()
cursor.close()
conn.close()
return result
try:
data = connect_to_database()
print("Data retrieved successfully:", data)
except Exception as e:
print(f"Failed to connect to the database: {e}")
Sample Output:
Error occurred: no such table: users. Retrying... Error occurred: no such table: users. Retrying... Error occurred: no such table: users. Retrying... Failed to connect to the database: Maximum retries exceeded. Function failed.
Explanation:
In the above exercise -
The function "connect_to_database()" uses the sqlite3 library to connect to a SQLite database file named example.db. The function executes a simple query to fetch all rows from the "users" table.
The "connect_to_database()" function is decorated with @retry_on_failure(max_retries=3, delay=2), which means it will be retried up to 3 times with a 2-second delay between retries if any exception occurs during execution.
The retry_on_failure decorator wraps the "connect_to_database()" function and provides retry logic. If an exception occurs during execution, the decorator will catch the exception, wait for the specified delay, and retry the function. When the maximum number of retries is reached and the function still fails, an exception is thrown.
Flowchart:
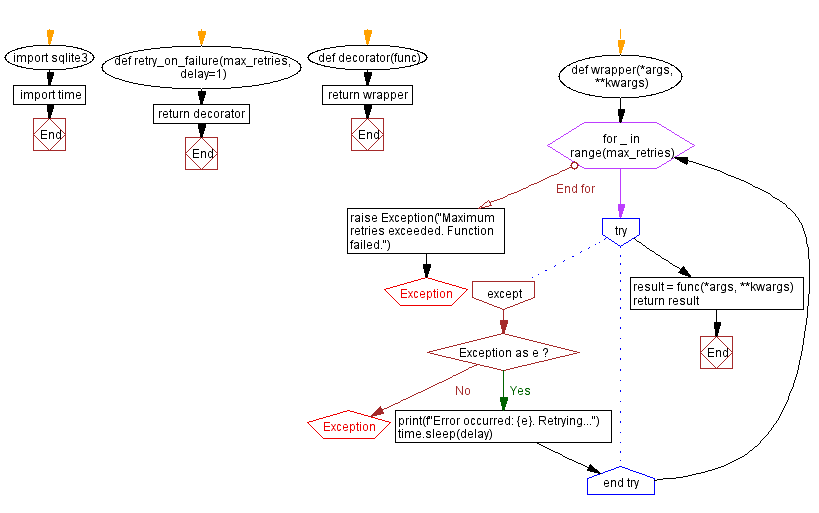
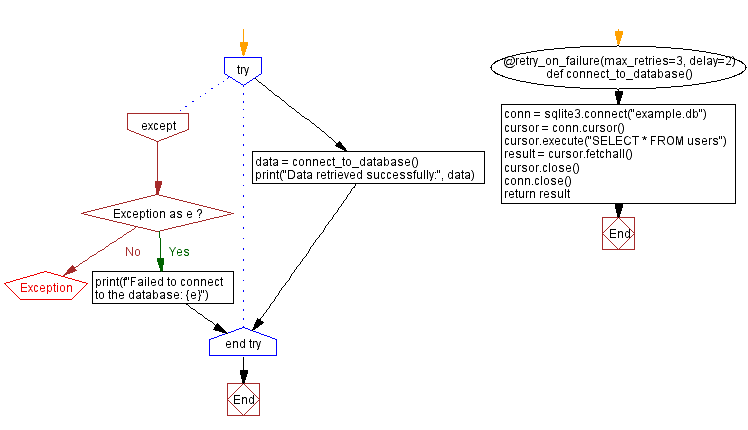
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Implementing a Python decorator for argument validation.
Next: Implementing a Python decorator for function rate limits.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics