Python logical AND and OR operations with Boolean values
Python Boolean Data Type: Exercise-2 with Solution
Write a Python function that takes two boolean values as input and returns the logical AND and OR of those values.
Sample Solution:
Code:
def logical_operations(x, y):
logical_and = x and y
logical_or = x or y
return logical_and, logical_or
def main():
try:
inputval1 = input("Input first boolean value (True/False): ").lower()
inputval2 = input("Input second boolean value (True/False): ").lower()
if inputval1 == "true":
bool1 = True
elif inputval1 == "false":
bool1 = False
else:
print("Invalid input for first boolean value.")
return
if inputval2 == "true":
bool2 = True
elif inputval2 == "false":
bool2 = False
else:
print("Invalid input for second boolean value.")
return
logical_and, logical_or = logical_operations(bool1, bool2)
print(f"Logical AND: {logical_and}")
print(f"Logical OR: {logical_or}")
except ValueError:
print("Invalid input. Please input either 'True' or 'False'.")
if __name__ == "__main__":
main()
Output:
Input first boolean value (True/False): FALSE Input second boolean value (True/False): TRUE Logical AND: False Logical OR: True
Input first boolean value (True/False): FALSE Input second boolean value (True/False): FALSE Logical AND: False Logical OR: False
Input first boolean value (True/False): TRUE Input second boolean value (True/False): TRUE Logical AND: True Logical OR: True
Input first boolean value (True/False): TRUE Input second boolean value (True/False): FALSE Logical AND: False Logical OR: True
The above exercise takes two boolean values as user input (either "True" or "False"), converts them to boolean variables, calculates their logical AND and OR using the "logical_operations()" function, and then prints the results.
Flowchart:
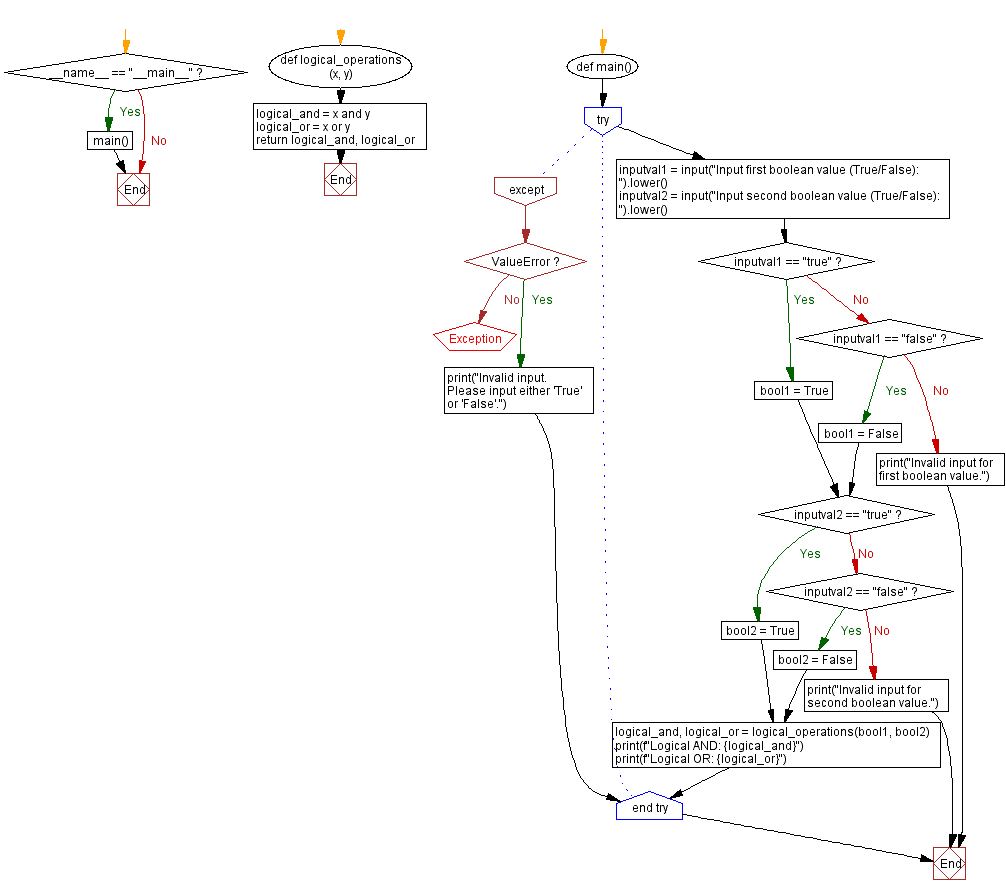
Previous: Check even or odd number using Boolean variables in Python.
Next: Voting eligibility checker.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics