Python circle area check using Boolean logic
Python Boolean Data Type: Exercise-9 with Solution
Write a Python program that creates a function that determines if a given point (x, y) is within a specified circle area using boolean conditions.
Sample Solution:
Code:
import math
def check_point_in_circle(x, y, center_x, center_y, radius):
distance = math.sqrt((x - center_x)**2 + (y - center_y)**2)
return distance <= radius
def main():
try:
center_x = float(input("Input x-coordinate of the circle center: "))
center_y = float(input("Input y-coordinate of the circle center: "))
radius = float(input("Input the radius of the circle: "))
point_x = float(input("Input x-coordinate of the point: "))
point_y = float(input("Input y-coordinate of the point: "))
if check_point_in_circle(point_x, point_y, center_x, center_y, radius):
print("The point is within the circle area.")
else:
print("The point is not within the circle area.")
except ValueError:
print("Invalid input. Please Input valid coordinates and radius.")
if __name__ == "__main__":
main()
Output:
Input x-coordinate of the circle center: 10 Input y-coordinate of the circle center: 10 Input the radius of the circle: 50 Input x-coordinate of the point: 2 Input y-coordinate of the point: 3 The point is within the circle area.
Input x-coordinate of the circle center: 5 Input y-coordinate of the circle center: 4 Input the radius of the circle: 4 Input x-coordinate of the point: 8 Input y-coordinate of the point: 9 The point is not within the circle area.
In the exercise above the program prompts the user to input the coordinates of the circle's center, the radius of the circle, and the coordinates of the point. Based on the distance between the point and the circle center, "is_point_in_circle()" determines if the point is within the circle area. It prints the appropriate message based on the results.
Flowchart:
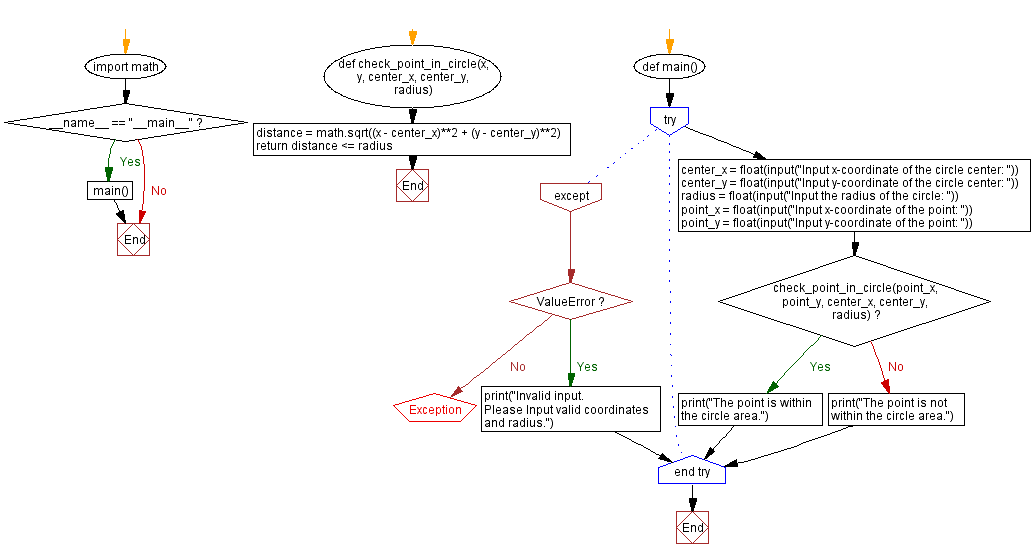
Previous:Python email validation using Boolean logic.
Next: Python user authentication using Boolean logic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics