Python Function: Printing arguments with *args
Python ellipsis (...) Data Type: Exercise-1 with Solution
Write a Python function that takes an arbitrary number of arguments using *args and prints each argument. Use ... to represent unspecified arguments.
Sample Solution:
Code:
# Define the ellipsis constant
var1 = ...
def print_arguments(*args):
"""
Prints each argument passed to the function, using var1 for unspecified arguments.
Args:
*args: Any number of positional arguments.
"""
for i, arg in enumerate(args):
print(f"Argument {i + 1}: {arg}")
if not args:
print(f"\nUnspecified arguments: {var1}")
# Example usage:
print_arguments(1, 2, 3, "Python")
print_arguments(50, [1, 2, 3], "Derya Nidia", "Mitul Calixta")
print_arguments() # Unspecified arguments: Ellipsis
Output:
Argument 1: 1 Argument 2: 2 Argument 3: 3 Argument 4: Python Argument 1: 50 Argument 2: [1, 2, 3] Argument 3: Derya Nidia Argument 4: Mitul Calixta Unspecified arguments: Ellipsis
In this code, 'var1' is defined as ..., and it is used to represent unspecified arguments when there are none provided to the function. If there are no arguments passed to the function, the code will print "Unspecified arguments: Ellipsis".
Flowchart:
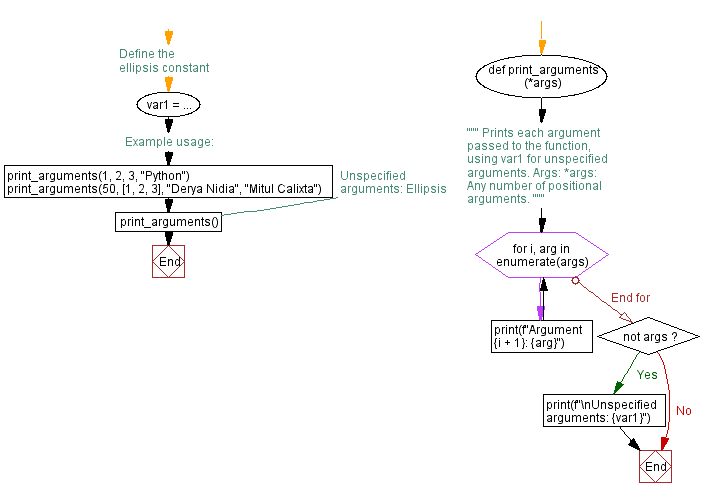
Previous: Python Extended Data Type ellipsis (...) Exercises Home.
Next: Python Function: Calling functions with flexible arguments.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics