Creating Python classes with dynamic attributes
Python ellipsis (...) Data Type: Exercise-9 with Solution
Write a Python program that creates a custom class with an 'init' method. Use 'ellipsis' to indicate that some attributes may be added dynamically.
Sample Solution:
Code:
class MyClass:
def __init__(self, attribute1, attribute2, attribute3, attribute4=...):
self.attribute1 = attribute1
self.attribute2 = attribute2
self.attribute3 = attribute3
self.attribute4 = attribute4
def set_attribute4(self, value):
self.attribute4 = value
# Create an instance of the MyClass
obj = MyClass("Red", "Green", "White")
# Access and print the attributes
print("Attribute 1:", obj.attribute1)
print("Attribute 2:", obj.attribute2)
print("Attribute 3:", obj.attribute3)
print("Attribute 4:", obj.attribute4) # Attribute 4 is represented by '...'
# Dynamically add a value to attribute3
obj.set_attribute4("Orange")
# Access and print the modified attribute3
print("Modified Attribute 4:", obj.attribute4)
Output:
Attribute 1: Red Attribute 2: Green Attribute 3: White Attribute 4: Ellipsis Modified Attribute 4: Orange
In the exercise above, we define the "MyClass" class with an 'init' method that takes three required attributes ('attribute1', 'attribute2' and 'attribute3') and one optional attribute ('attribute4') represented by 'ellipsis (...)'. This indicates that 'attribute4' may or may not be provided during object creation.
We create an instance of the "MyClass" class and access its attributes. Initially, 'attribute4' is represented by '...' . Later, we dynamically set a value for 'attribute4' using the "set_attribute4()" method.
Flowchart:
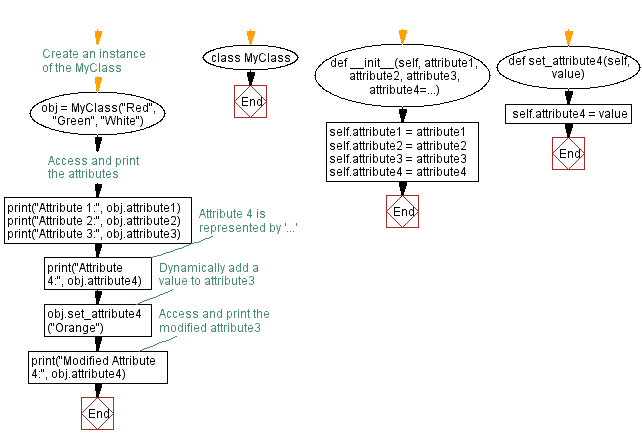
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics