Python OrderedDict sorting: Keys and values
Python OrderedDict Data Type: Exercise-2 with Solution
Write a Python program that sorts the OrderedDict by its keys. Sort the OrderedDict by its values as well.
Sample Solution:
Code:
from collections import OrderedDict
# Create an OrderedDict
ordered_dict = OrderedDict()
ordered_dict['Laptop'] = 40
ordered_dict['Desktop'] = 45
ordered_dict['Mobile'] = 35
# Print the OrderedDict contents
print("OrderedDict contents:")
for item, quantity in ordered_dict.items():
print(f"Itemt: {item}, Quantity: {quantity}")
# Sort OrderedDict by keys
sorted_by_keys = OrderedDict(sorted(ordered_dict.items(), key=lambda item: item[0]))
# Sort OrderedDict by values
sorted_by_values = OrderedDict(sorted(ordered_dict.items(), key=lambda item: item[1]))
# Print sorted by keys
print("\nSorted by keys:")
for item, quantity in sorted_by_keys.items():
print(f"Item: {item}, Quantity: {quantity}")
# Print sorted by values
print("\nSorted by values:")
for item, quantity in sorted_by_values.items():
print(f"Item: {item}, Quantity: {quantity}")
Output:
OrderedDict contents: Itemt: Laptop, Quantity: 40 Itemt: Desktop, Quantity: 45 Itemt: Mobile, Quantity: 35 Sorted by keys: Item: Desktop, Quantity: 45 Item: Laptop, Quantity: 40 Item: Mobile, Quantity: 35 Sorted by values: Item: Mobile, Quantity: 35 Item: Laptop, Quantity: 40 Item: Desktop, Quantity: 45
In the exercise above, we use the "sorted()" function to sort the OrderedDict items. For sorting by keys, we provide a lambda function that extracts the key from each item. For sorting by values, we provide another lambda function that extracts the value from each item. Sorted items are then used to create new OrderedDict instances.
Flowchart:
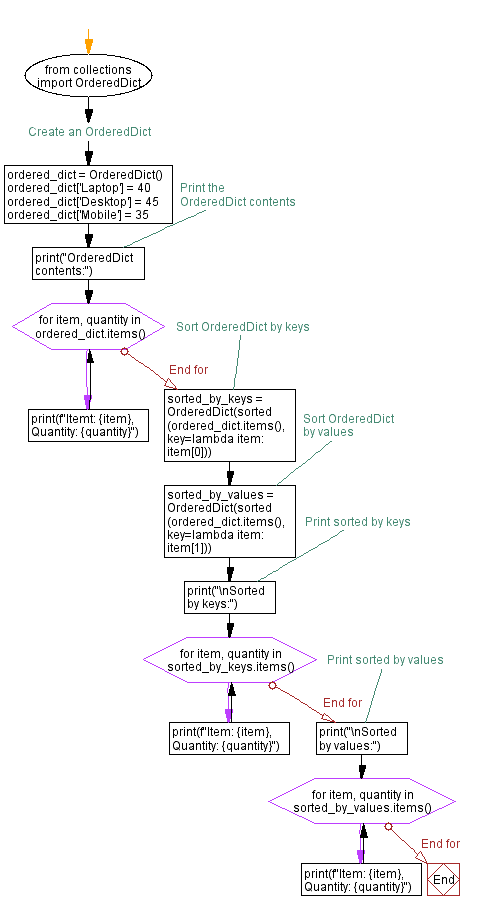
Previous: Python OrderedDict example: Adding items and printing contents.
Next: Python OrderedDict access and existence check.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics