Python OrderedDict access and existence check
Python OrderedDict Data Type: Exercise-3 with Solution
Write a Python program that accesses an item in the OrderedDict by its key. Check if a specified item exists in the OrderedDict as well.
Sample Solution:
Code:
from collections import OrderedDict
# Create an OrderedDict
ordered_dict = OrderedDict()
ordered_dict['Laptop'] = 40
ordered_dict['Desktop'] = 45
ordered_dict['Mobile'] = 35
# Access an item by its key
item = ordered_dict['Desktop']
print(f"Quantity of Desktops: {item}")
# Check if a specified item exists
item_to_check = 'Mobile'
if item_to_check in ordered_dict:
print(f"{item_to_check} exists in the OrderedDict")
else:
print(f"{item_to_check} does not exist in the OrderedDict")
# Check if a specified item exists
item_to_check = 'Charger'
if item_to_check in ordered_dict:
print(f"{item_to_check} exists in the OrderedDict")
else:
print(f"{item_to_check} does not exist in the OrderedDict")
Output:
Quantity of Desktops: 45 Mobile exists in the OrderedDict Charger does not exist in the OrderedDict
In the exercise above, we use 'square brackets' to access an item in the OrderedDict using its key ('Desktop'). We also use the 'in' keyword to check if a specified item ('Mobile' / 'Charger') exists in the OrderedDict.
Flowchart:
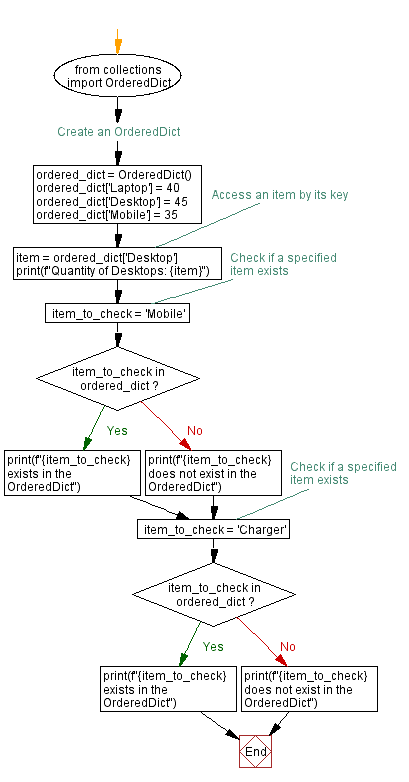
Previous: Python OrderedDict sorting: Keys and values.
Next: Python OrderedDict Reversal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics