Python OrderedDict Key-Value removal
Python OrderedDict Data Type: Exercise-6 with Solution
Write a Python program to create an OrderedDict with the following key-value pairs:
'Laptop': 40
'Desktop': 45
'Mobile': 35
'Charger': 25
Now remove the first key-value pair and print the updated OrderedDict.
Sample Solution:
Code:
from collections import OrderedDict
# Create an OrderedDict with the given key-value pairs
ordered_dict = OrderedDict([
('Laptop', 40),
('Desktop', 45),
('Mobile', 35),
('Charger', 25)
])
print("Original OrderedDict:")
print(ordered_dict)
# Remove the first key-value pair
print("\nRemove the first key-value pair of the said OrderedDict:")
ordered_dict.popitem(last=False)
# Print the updated OrderedDict
print("\nUpdated OrderedDict:")
print(ordered_dict)
Output:
Original OrderedDict: OrderedDict([('Laptop', 40), ('Desktop', 45), ('Mobile', 35), ('Charger', 25)]) Remove the first key-value pair of the said OrderedDict: Updated OrderedDict: OrderedDict([('Desktop', 45), ('Mobile', 35), ('Charger', 25)])
In the exercise above, the popitem(last=False) method of the OrderedDict is used to remove the first key-value pair (with the lowest index) from the dictionary. The last parameter is set to False to indicate that the item should be removed from the beginning of the dictionary.
Flowchart:
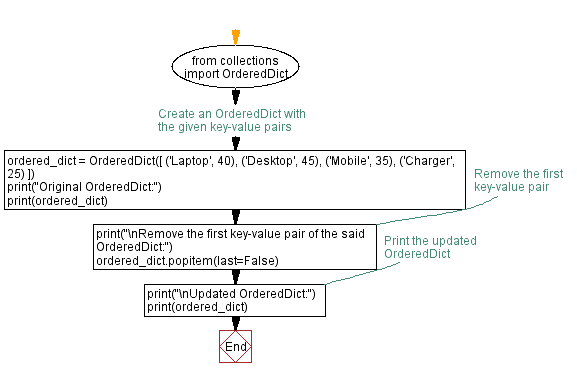
Previous: Python OrderedDict Key reordering.
Next: Python merging OrderedDicts with summed values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics