Python function to handle empty strings
Python None Data Type: Exercise-1 with Solution
Write a Python function that takes a string as input and returns "None" if the string is empty, otherwise it returns the given string.
Sample Solution:
Code:
def check_string(input_string):
if not input_string:
return "None"
return input_string
def main():
try:
input_string = input("Input a string: ")
result = check_string(input_string)
print("Result:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a string: Result: None
Input a string: python Result: python
In the above exercise the program defines the "check_string()" function, which checks if the input string is empty. If it is, it returns the string "None", otherwise, it returns the original input string. The "main()" function takes user input, processes it using the "check_string()" function, and prints the result.
Flowchart:
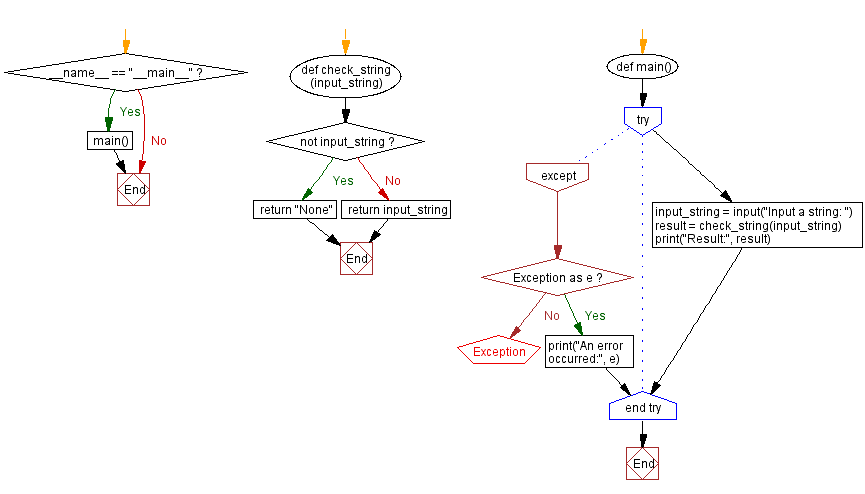
Previous: Python Extended Data Type None Exercises Home.
Next: Python function to retrieve middle character
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics