Python function to retrieve middle character
Python None Data Type: Exercise-2 with Solution
Write a Python function that returns the middle character of a string or "None" if the string length is odd.
Sample Solution:
Code:
def get_middle_character(input_string):
length = len(input_string)
if length % 2 == 0:
return "None"
else:
middle_index = length // 2
return input_string[middle_index]
def main():
try:
input_string = input("Input a string: ")
result = get_middle_character(input_string)
if result == "None":
print("String length is even, no middle character.")
else:
print("Middle character:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a string: abcd String length is even, no middle character.
Input a string: abcde Middle character: c
In the above exercise, the program defines the "get_middle_character()" function, which calculates the length of the input string and checks if it's odd. If it's odd, it returns the middle character of the string; otherwise, it returns "None". In the "main()" function, user input is processed using "get_middle_character()", and the result is printed.
Flowchart:
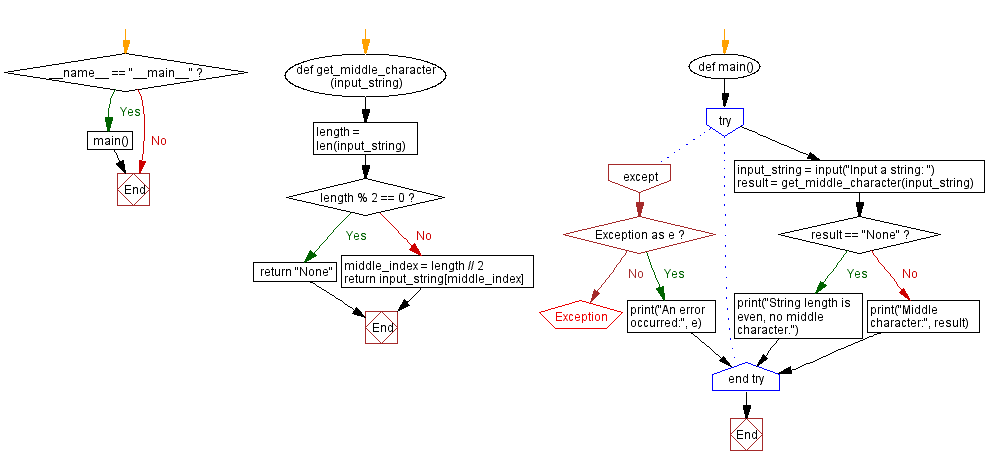
Previous: Python function to handle empty strings.
Next: Python program for handling empty strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics