Python program for handling empty strings
Python None Data Type: Exercise-3 with Solution
Write a Python program that iterates through a list of strings and prints each string. If a string is empty, print "Empty string" instead.
Sample Solution:
Code:
def empty_string_handling(string_list):
for s in string_list:
if s:
print(s)
else:
print(None)
def main():
try:
text_list = ["Python", "", "Java", "C++", "", "C#"]
empty_string_handling(text_list)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Python None Java C++ None C#
In the exercise above, the "empty_string_handling()" function uses the boolean value of each string to decide whether to print the string itself or 'None'. If the string is not empty, it evaluates to 'True' and is printed. Otherwise, it evaluates to 'False' and 'None' is printed.
Flowchart:
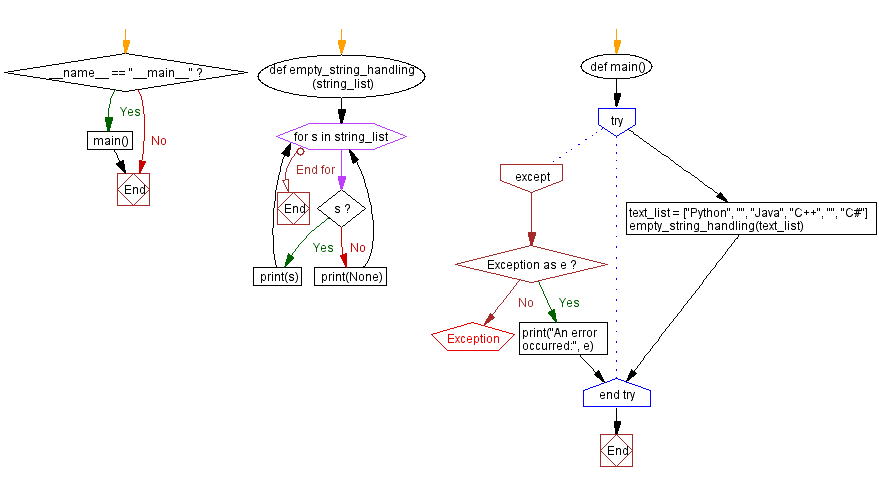
Previous: Python function to retrieve middle character.
Next: Check descending sort & empty list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics