Recursive Python function: Count None values in list
Python None Data Type: Exercise-7 with Solution
Write a Python function that counts None values in a list recursively. Return 0 if the list is empty.
Sample Solution:
Code:
def count_none(input_list):
if not input_list:
return 0
head, *tail = input_list
if head is None:
return 1 + count_none(tail)
else:
return count_none(tail)
def main():
try:
values_list = [None, "Red", None, 8, None, True]
result = count_none(values_list)
print("Number of None values:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Number of None values: 3
In the exercise above, the "count_none()" function uses recursion to process the input list. It checks if the list is empty; if it is, it returns 0. Alternatively, it separates the first element (head) from the rest (tail). In case head is 'None', it increments the count and calls the function recursively with the remaining tail. If head is not 'None', it calls the function recursively with the remaining tail. The "main()" function demonstrates the function's usage by providing a sample list of values.
Flowchart:
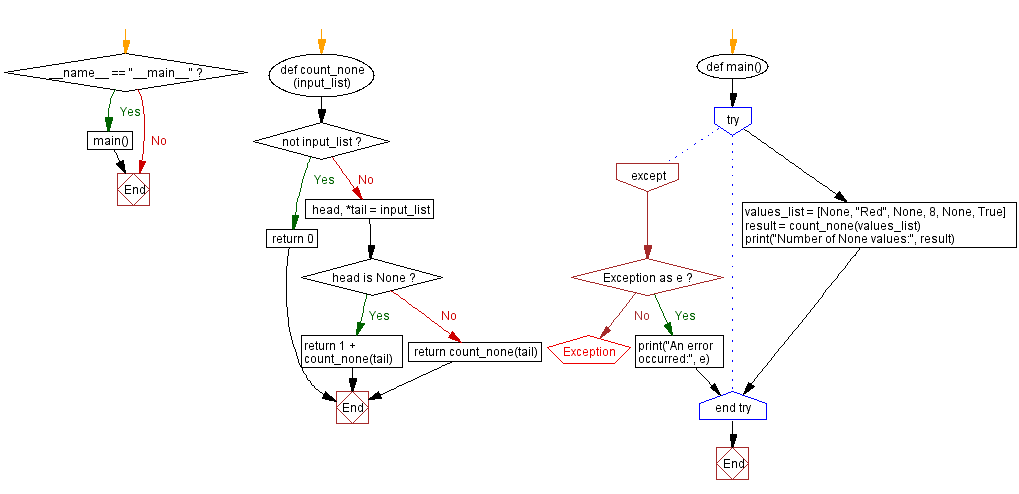
Previous: Python dictionary value retrieval with key check.
Next: Sum with None check
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics