Python Function: Sum with None check
Python None Data Type: Exercise-8 with Solution
Write a Python function that takes two arguments and returns their sum if both aren't None, otherwise return None.
Sample Solution:
Code:
def sum_without_none(a, b):
if a is not None and b is not None:
return a + b
else:
return None
def main():
try:
num1 = 3
num2 = None
result = sum_without_none(num1, num2)
if result is None:
print("At least one argument is None.")
else:
print("Sum:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Sum: 10
At least one argument is None
In the exercise above, the "sum_without_none()" function checks if both arguments 'x' and 'y' are not 'None'. If they are not 'None', it returns their sum; otherwise, it returns None. The main function demonstrates the function's usage by providing two sample numbers. It prints the sum if both numbers are not 'None', or indicates that at least one argument is 'None'.
Flowchart:
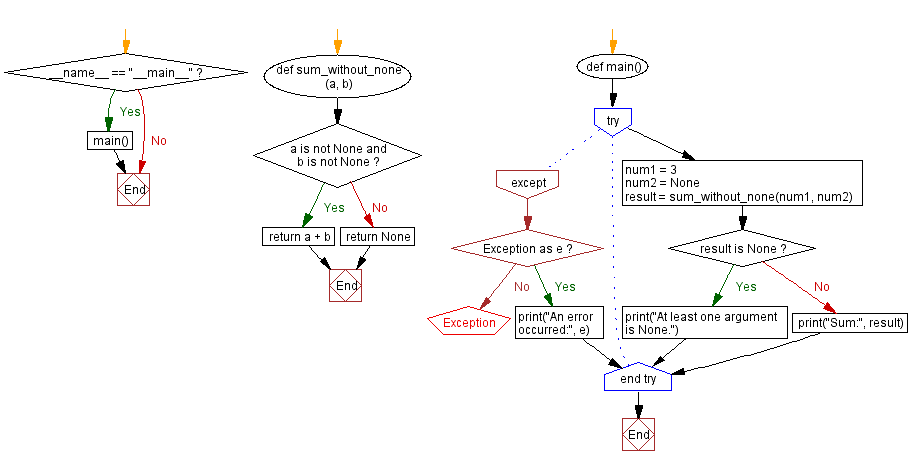
Previous: Count None values in list.
Next: Insert None between list elements
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics