Python Happy number generator: Generate the next happy number
Python: Generators Yield Exercise-12 with Solution
Write a Python program to implement a generator that generates the next happy number after a given number.
From Wikipedia,
In number theory, a happy number is a number which eventually reaches 1 when replaced by the sum of the square of each digit. For instance, 13 is a happy number because 12 + 32 = 10 and 12 + o2 = 1
On the other hand, 4 is not a happy number because the sequence starting with 42 = 16 and 12 + 62 = 37 eventually reaches 22 + 02 =4, the number that started the sequence, and so the process continues in an infinite cycle without ever reaching 1. A number which is not happy is called sad or unhappy.
The first few happy numbers are 1, 7, 10, 13, 19, 23, 28, 31, 32, 44, 49, 68, 70, 79, 82, 86, 91, 94, 97, 100, ...
Sample Solution:
Python Code:
def is_happy_number(num):
visited = set()
while True:
if num == 1:
return True
elif num in visited:
return False
visited.add(num)
num = sum(int(digit) ** 2 for digit in str(num))
def next_happy_number(start):
num = start + 1
while True:
if is_happy_number(num):
yield num
num += 1
# Accept a positive number from the user
start_num = int(input("Input a number(positive): "))
# Create the next happy number generator
happy_num_gen = next_happy_number(start_num)
# Generate and print the next happy number
print("Next happy number after", start_num, "is:")
print(next(happy_num_gen))
Sample Output:
Input a number(positive): 7 Next happy number after 7 is: 10
Input a number(positive): 31 Next happy number after 31 is: 32
Input a number(positive):70 Next happy number after 70 is: 79
Explanation:
In the above exercise -
- The "is_happy_number()" function determines whether a given number is a happy number or not. During the calculation, a set called visited keeps track of visited numbers. It continuously calculates the sum of the squares of the digits until the sum becomes 1 or it encounters the sum again.
- The next_happy_number generator function generates the next happy number after a given number. It starts with start + 1 and iterates indefinitely. It checks whether each number is a happy number using the is_happy_number function. If it is, it yields the number.
- The program accepts a number from the user as the starting number. It then creates the next_happy_number generator using the input number. Finally, it generates and prints the next happy number after the starting number.
Flowchart:
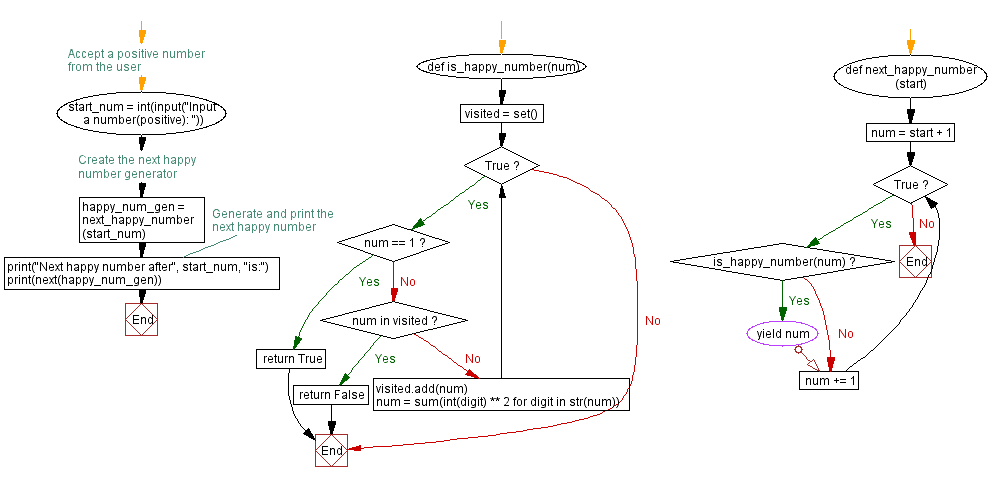
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Generate all string permutations.
Next: Generate square, cube roots of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics