NumPy: Create a 2-dimensional array of size 2 x 3
NumPy: Array Object Exercise-37 with Solution
Write a NumPy program to create a 2-dimensional array of size 2 x 3 (composed of 4-byte integer elements), also print the shape, type and data type of the array.
Pictorial Presentation:
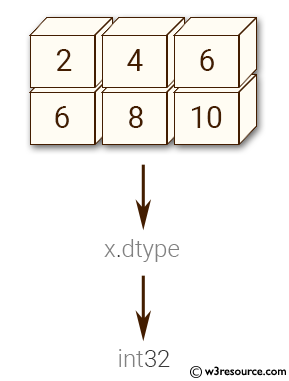
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 2D NumPy array with two rows and three columns with data type np.int32
x = np.array([[2, 4, 6], [6, 8, 10]], np.int32)
# Checking and displaying the type of variable 'x'
print(type(x))
# Displaying the shape of the NumPy array 'x' (number of rows, number of columns)
print(x.shape)
# Displaying the data type of the elements in the array 'x'
print(x.dtype)
Sample Output:
<class 'numpy.ndarray'> (2, 3) int32
Explanation:
In the above code -
x = np.array([[2, 4, 6], [6, 8, 10]], np.int32): The current line creates a two-dimensional NumPy array x with two rows and three columns using the provided list of lists. The data type of the array is specified as np.int32 (32-bit integers).
print(type(x)): The current line prints the type of the ‘x’ array, which is <class 'numpy.ndarray'>.
print(x.shape): The current line prints the shape of the ‘x’ array, which is (2, 3) - two rows and three columns.
print(x.dtype): The current line prints the data type of the elements in the ‘x’ array, which is int32, as specified during the array creation.
Python-Numpy Code Editor:
Previous: Write a NumPy program to create a contiguous flattened array.
Next: Write a NumPy program to create a new shape to an array without changing its data.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics