Python PyQt slider application
Python PyQt Connecting Signals to Slots: Exercise-4 with Solution
Write a Python program that creates a sliding bar on the screen. The slider will display a number that shows where it is. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QSlider Class: The QSlider widget provides a vertical or horizontal slider.
QLabel Class: The QLabel widget provides a text or image display.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QSlider, QLabel, QVBoxLayout
from PyQt5.QtCore import Qt
class SliderApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Slider App")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
layout = QVBoxLayout()
# Create a QSlider widget
self.slider = QSlider(Qt.Horizontal, self)
layout.addWidget(self.slider)
# Create a QLabel widget
self.label = QLabel("Slider Value: 0", self)
layout.addWidget(self.label)
# Connect the slider's valueChanged signal to the update_label slot
self.slider.valueChanged.connect(self.update_label)
self.setLayout(layout)
# Slot function to update the label with the current slider value
def update_label(self):
value = self.slider.value()
self.label.setText(f"Slider Value: {value}")
def main():
app = QApplication(sys.argv)
window = SliderApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
Import the necessary modules from PyQt5.
Create a custom "QWidget" class named "SliderApp", which contains the entire application logic.
Inside the "SliderApp" class, we create a horizontal QSlider widget (self.slider) and a QLabel widget (self.label) for displaying the slider's current value.
Connect the "valueChanged" signal of the slider to the update_label slot function using self.slider.valueChanged.connect(self.update_label).
The "update_label()" slot function retrieves the current slider value using self.slider.value() and updates the text of the "QLabel" to display the current slider value.
In the main function, we create the PyQt application, instantiate the "SliderApp" class, and show the main window.
Output:
Flowchart:
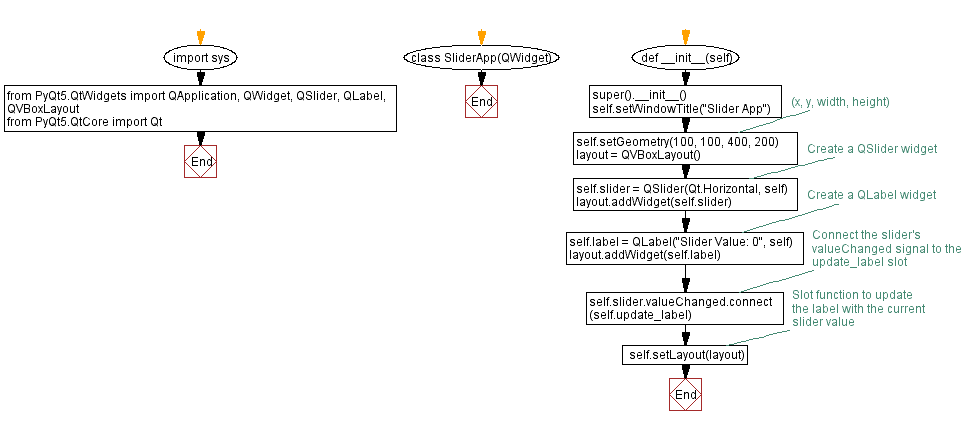
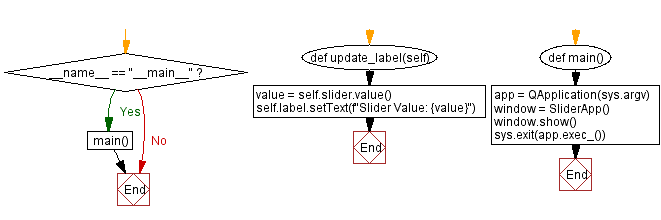
Python Code Editor:
Previous: Python PyQt text updater application.
Next: Python PyQt comboBox example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics