Python PyQt comboBox example
Write a Python program that creates a combo box with different items. When you select an item from the list, it should appear somewhere on the screen immediately. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QComboBox Class: The QComboBox widget is a combined button and popup list.
QLabel Class: The QLabel widget provides a text or image display.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QComboBox, QLabel, QVBoxLayout
def main():
app = QApplication(sys.argv)
window = QWidget()
window.setWindowTitle("Combo Box Example")
window.setGeometry(100, 100, 400, 200) # (x, y, width, height)
layout = QVBoxLayout()
# Create a QComboBox widget
combo_box = QComboBox(window)
combo_box.addItem("Python")
combo_box.addItem("Java")
combo_box.addItem("C++")
layout.addWidget(combo_box)
# Create a QLabel to display the selected item
label = QLabel("Selected Item: ", window)
layout.addWidget(label)
# Connect the combo box's currentIndexChanged signal to update_label slot
def update_label():
selected_item = combo_box.currentText()
label.setText("Selected Item: " + selected_item)
combo_box.currentIndexChanged.connect(update_label)
window.setLayout(layout)
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a PyQt application and a main window.
- Create a "QComboBox" widget called combo_box and add three items to it.
- Create a "QLabel" widget called label to display the selected item.
- Define a slot function called "update_label()" that updates the label text with the currently selected item from the combo box.
- Connect the "currentIndexChanged" signal of the combo box to the "update_label()" slot function, so that the label is updated whenever the selection changes.
- At last, we display the PyQt application's main window and start its event loop.
Output:
Flowchart:
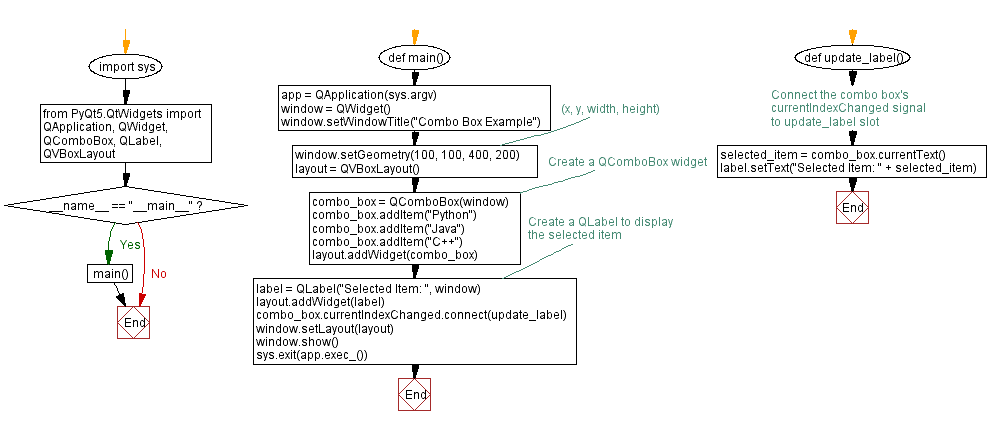
Go to:
Previous: Python PyQt slider application.
Next: Python PyQt button click example.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.