Python PyQt character counter notepad
Python PyQt Connecting Signals to Slots: Exercise-8 with Solution
Write a Python program that creates a notepad system where you can type, edit and display text. As you write, it should automatically count and show how many characters you've written. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QTextEdit Class: The QTextEdit class provides a widget that is used to edit and display both plain and rich text.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QLabel Class: The QLabel widget provides a text or image display.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QTextEdit, QVBoxLayout, QLabel
def main():
app = QApplication(sys.argv)
window = QWidget()
window.setWindowTitle("Character Counter")
window.setGeometry(100, 100, 400, 200) # (x, y, width, height)
layout = QVBoxLayout()
# Create a QTextEdit widget for text input
text_edit = QTextEdit(window)
layout.addWidget(text_edit)
# Create a QLabel to display the character count
char_count_label = QLabel("Character Count: 0", window)
layout.addWidget(char_count_label)
# Connect the textChanged signal of the QTextEdit to a slot that updates the character count
def update_char_count():
char_count = len(text_edit.toPlainText())
char_count_label.setText(f"Character Count: {char_count}")
text_edit.textChanged.connect(update_char_count)
window.setLayout(layout)
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a PyQt application and a main window.
- Design a "QTextEdit" widget called text_edit to allow text input.
- Create a "QLabel" widget called 'char_count_label' to display the character count.
- Define a slot function called "update_char_count()" that calculates the character count in "QTextEdit" and updates the label text accordingly.
- Connect the textChanged signal of "QTextEdit" to the "update_char_count()" slot function.
- Finally, we set up the layout, display the main window, and start the PyQt application's event loop.
Output:
Flowchart:
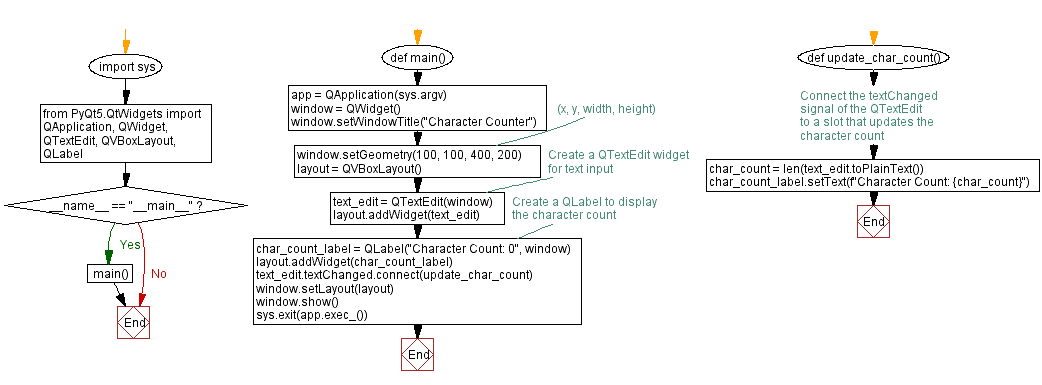
Python Code Editor:
Previous: Python PyQt custom signal example.
Next: Python PyQt simple calculator.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics