Python SQLite database with Tkinter
Python Tkinter File Operations and Integration: Exercise-11 with Solution
Write a Python program to create, read, and delete data from an SQLite database within a Tkinter application.
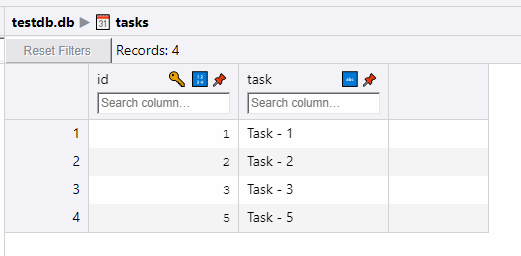
Sample Solution:
Python Code:
import tkinter as tk
import sqlite3
from tkinter import messagebox
class DatabaseApp:
def __init__(self, root):
self.root = root
self.root.title("SQLite Database Example")
# Create a database or connect to an existing one
self.conn = sqlite3.connect("testdb.db")
self.cursor = self.conn.cursor()
# Create a table if it doesn't exist
self.cursor.execute('''CREATE TABLE IF NOT EXISTS tasks (id INTEGER PRIMARY KEY, task TEXT)''')
self.conn.commit()
# Create GUI elements
self.task_label = tk.Label(root, text="Task:")
self.task_label.pack()
self.task_entry = tk.Entry(root)
self.task_entry.pack()
self.add_button = tk.Button(root, text="Add Task", command=self.add_task)
self.add_button.pack()
self.task_listbox = tk.Listbox(root)
self.task_listbox.pack()
self.delete_button = tk.Button(root, text="Delete Task", command=self.delete_task)
self.delete_button.pack()
self.load_tasks()
def add_task(self):
task = self.task_entry.get()
if task:
self.cursor.execute("INSERT INTO tasks (task) VALUES (?)", (task,))
self.conn.commit()
self.load_tasks()
self.task_entry.delete(0, tk.END)
else:
messagebox.showwarning("Warning", "Please input a task.")
def load_tasks(self):
self.task_listbox.delete(0, tk.END)
self.cursor.execute("SELECT * FROM tasks")
tasks = self.cursor.fetchall()
for row in tasks:
self.task_listbox.insert(tk.END, row[1])
def delete_task(self):
selected_task = self.task_listbox.get(tk.ACTIVE)
if selected_task:
self.cursor.execute("DELETE FROM tasks WHERE task=?", (selected_task,))
self.conn.commit()
self.load_tasks()
else:
messagebox.showwarning("Warning", "Please select a task to delete.")
def __del__(self):
self.conn.close()
if __name__ == "__main__":
root = tk.Tk()
app = DatabaseApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Create a database named "testdb.db" or connect to it if it already exists.
- Create a table named tasks to store tasks with unique IDs and description.
- The GUI allows us to add tasks, load tasks from the database, and delete selected tasks.
- Use the "sqlite3" library to execute SQL queries and interact with the SQLite database.
Output:
Flowchart:
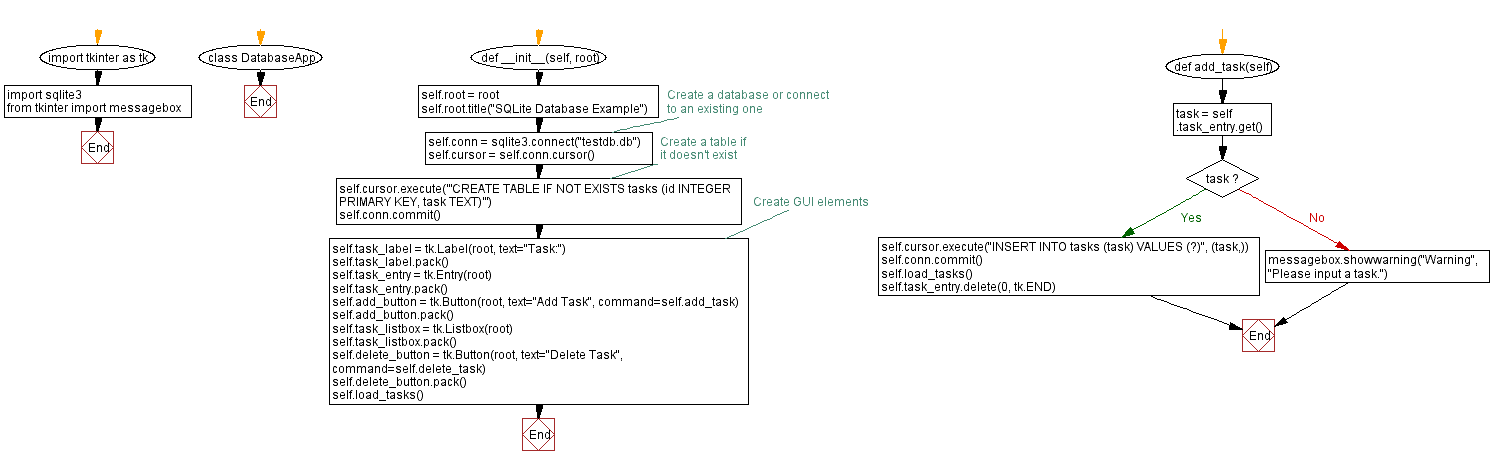
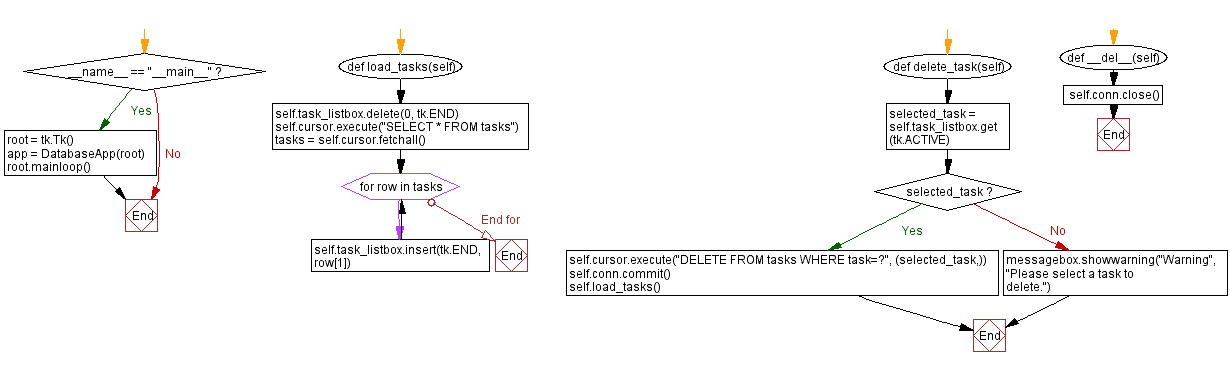
Python Code Editor:
Previous: Python Excel data viewer with Tkinter.
Next: Python Tkinter CRUD application with SQLite.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics