Python Tkinter CSV data entry and writer
Python Tkinter File Operations and Integration: Exercise-3 with Solution
Write a Python program that writes data to a CSV file using the Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
import csv
class CSVWriterApp:
def __init__(self, root):
self.root = root
self.root.title("CSV Writer")
# Create labels and entry fields for data input
self.label1 = tk.Label(root, text="Student Name:")
self.entry1 = tk.Entry(root)
self.label2 = tk.Label(root, text="Class:")
self.entry2 = tk.Entry(root)
self.label3 = tk.Label(root, text="Marks:")
self.entry3 = tk.Entry(root)
# Create a "Save" button
self.save_button = tk.Button(root, text="Save", command=self.save_data)
# Place labels, entry fields, and button on the grid
self.label1.grid(row=0, column=0)
self.entry1.grid(row=0, column=1)
self.label2.grid(row=1, column=0)
self.entry2.grid(row=1, column=1)
self.label3.grid(row=2, column=0)
self.entry3.grid(row=2, column=1)
self.save_button.grid(row=3, column=0, columnspan=2)
def save_data(self):
# Get data from entry fields
name = self.entry1.get()
age = self.entry2.get()
country = self.entry3.get()
# Open the CSV file for writing
with open("temp.csv", mode="a", newline="") as csv_file:
csv_writer = csv.writer(csv_file)
# Write the data to the CSV file
csv_writer.writerow([name, age, country])
# Clear the entry fields
self.entry1.delete(0, tk.END)
self.entry2.delete(0, tk.END)
self.entry3.delete(0, tk.END)
if __name__ == "__main__":
root = tk.Tk()
app = CSVWriterApp(root)
root.mainloop()
Explanation:
In the exercise above -
The program creates a GUI application using Tkinter where you can input data into input fields (Student Name, Class, Marks). When you click the "Save" button, it takes the input values and appends them as a new row to a CSV file named "temp.csv." The file name and path can be modified.
Output:
Flowchart:
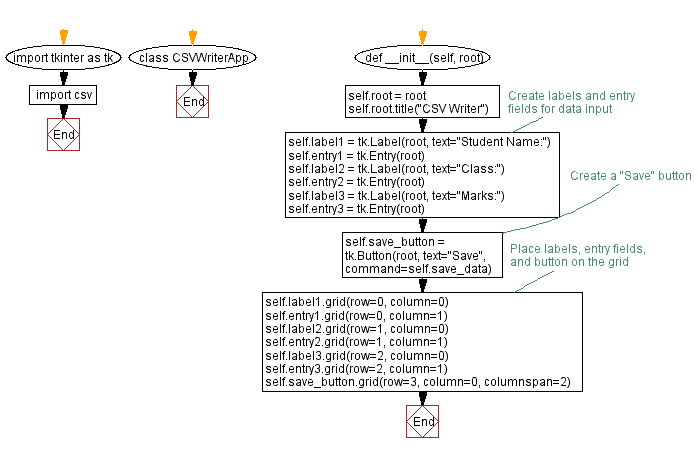
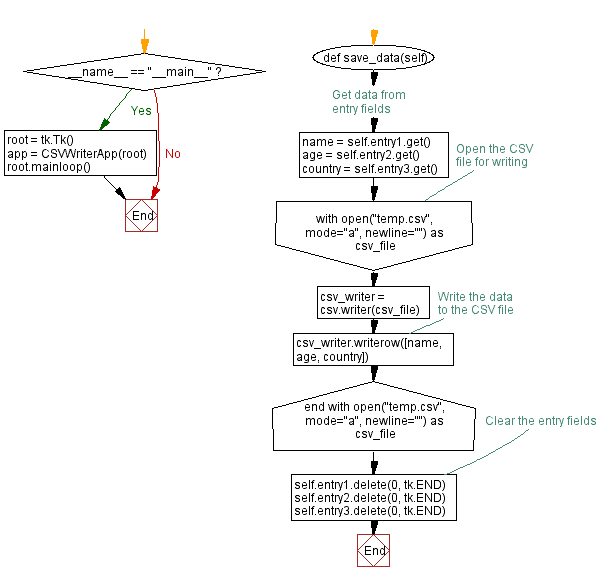
Python Code Editor:
Previous: Python Tkinter CSV viewer with tabular display.
Next: Python Tkinter JSON viewer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics