Python Tkinter Calendar App
Python Tkinter File Operations and Integration: Exercise-7 with Solution
Write a Python program that builds a calendar application using Tkinter. Allow users to view and edit appointments stored in text files.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
from tkinter import filedialog
# Function to open a text file
def open_file():
global current_file
current_file = filedialog.askopenfilename(filetypes=[("Text Files", "*.txt")])
load_appointments()
# Function to save appointments to the current text file
def save_file():
if current_file:
with open(current_file, 'w') as file:
for date, appointment in appointments.items():
file.write(f"{date}: {appointment}\n")
# Function to load appointments from the current text file
def load_appointments():
if current_file:
appointments.clear()
with open(current_file, 'r') as file:
for line in file:
parts = line.strip().split(': ')
if len(parts) == 2:
date, appointment = parts
appointments[date] = appointment
update_calendar()
# Function to add or edit an appointment
def add_edit_appointment():
date = date_var.get()
appointment = appointment_entry.get()
if date and appointment:
appointments[date] = appointment
update_calendar()
save_file()
clear_entry_fields()
# Function to clear date and appointment entry fields
def clear_entry_fields():
date_var.set('')
appointment_entry.delete(0, tk.END)
# Function to update the calendar display
def update_calendar():
calendar_display.config(state=tk.NORMAL)
calendar_display.delete(1.0, tk.END)
for date, appointment in sorted(appointments.items()):
calendar_display.insert(tk.END, f"{date}: {appointment}\n")
calendar_display.config(state=tk.DISABLED)
# Create the main window
root = tk.Tk()
root.title("Calendar App")
# Create a Frame for file operations
file_frame = ttk.LabelFrame(root, text="File")
file_frame.pack(fill=tk.BOTH, expand=True, padx=10, pady=5)
# Open file button
open_button = ttk.Button(file_frame, text="Open File", command=open_file)
open_button.pack(side=tk.LEFT, padx=5)
# Save file button
save_button = ttk.Button(file_frame, text="Save File", command=save_file)
save_button.pack(side=tk.LEFT, padx=5)
# Create a Frame for adding/editing appointments
add_edit_frame = ttk.LabelFrame(root, text="Add/Edit Appointment")
add_edit_frame.pack(fill=tk.BOTH, expand=True, padx=10, pady=5)
# Date entry
date_label = ttk.Label(add_edit_frame, text="Date:")
date_label.grid(row=0, column=0, padx=5, pady=5)
date_var = tk.StringVar()
date_entry = ttk.Entry(add_edit_frame, textvariable=date_var)
date_entry.grid(row=0, column=1, padx=5, pady=5)
# Appointment entry
appointment_label = ttk.Label(add_edit_frame, text="Appointment:")
appointment_label.grid(row=1, column=0, padx=5, pady=5)
appointment_entry = ttk.Entry(add_edit_frame)
appointment_entry.grid(row=1, column=1, padx=5, pady=5)
# Add/Edit button
add_edit_button = ttk.Button(add_edit_frame, text="Add/Edit", command=add_edit_appointment)
add_edit_button.grid(row=2, columnspan=2, padx=5, pady=10)
# Create a Frame for displaying appointments
calendar_frame = ttk.LabelFrame(root, text="Calendar")
calendar_frame.pack(fill=tk.BOTH, expand=True, padx=10, pady=5)
# Calendar display
calendar_display = tk.Text(calendar_frame, wrap=tk.WORD, state=tk.DISABLED)
calendar_display.pack(fill=tk.BOTH, expand=True, padx=5, pady=5)
# Initialize global variables
current_file = None
appointments = {}
# Main loop
root.mainloop()
Explanation:
In the exercise above -
- You can add or edit appointments by specifying a date and appointment description.
- Appointments are displayed in a text widget, which also provides read-only viewing.
- The program provides options to load appointments from a text file, save appointments to the same file, and display, add, or edit appointments.
- When you open a file, the program reads appointments from the file and displays them on the calendar display. When you add or edit appointments (using dates), the changes are updated in the text file.
Output:
Flowchart:
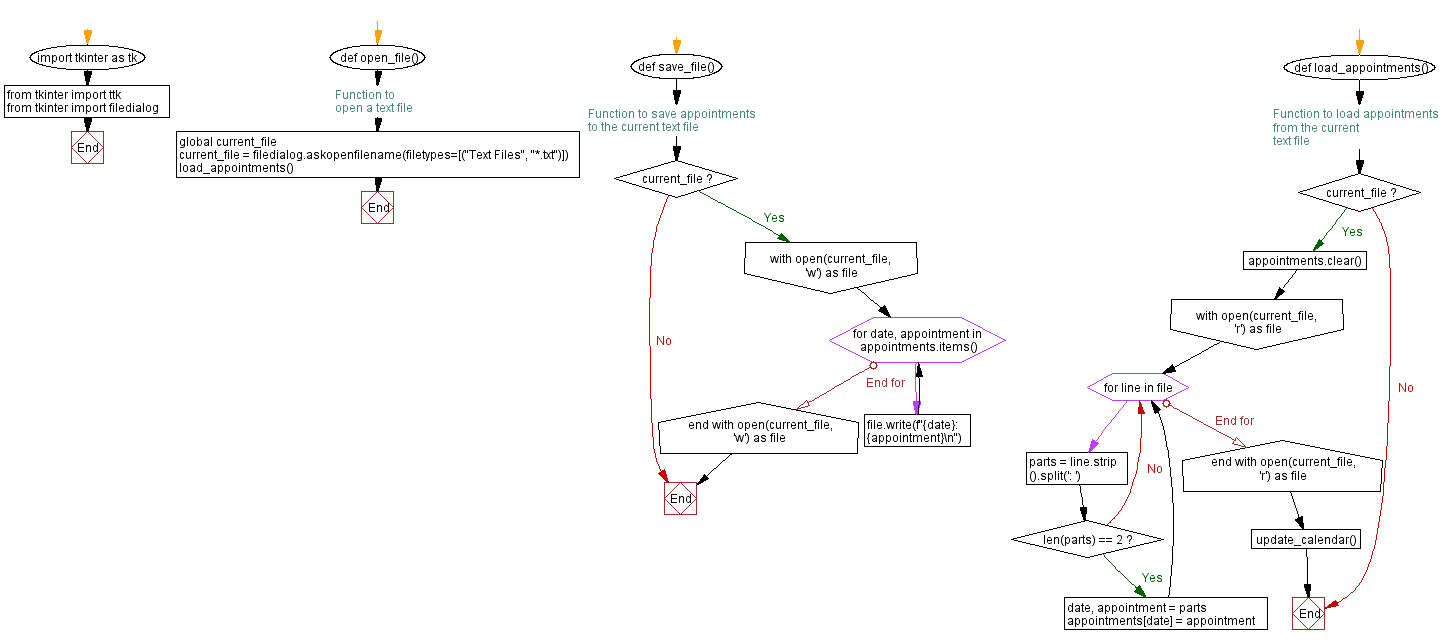
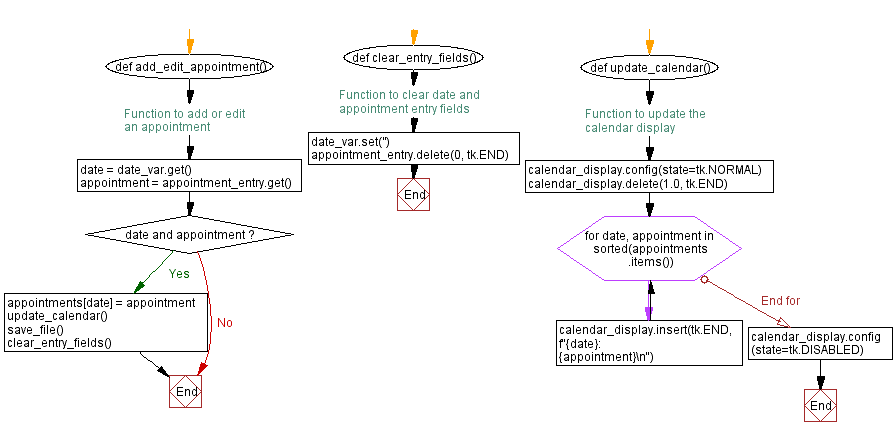
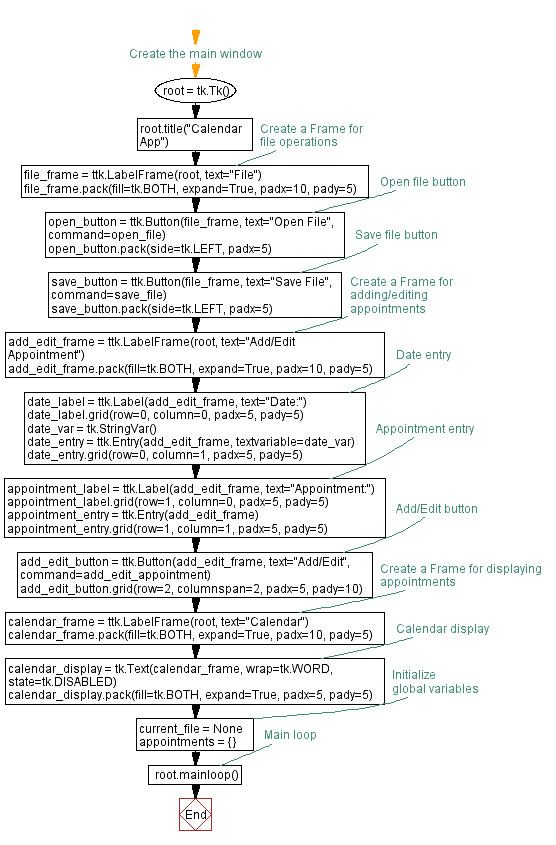
Python Code Editor:
Previous: Python Tkinter log viewer.
Next: Python Excel data viewer with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics