Python Excel data viewer with Tkinter
Python Tkinter File Operations and Integration: Exercise-8 with Solution
Write a Python program that reads and displays data from an Excel spreadsheet using the openpyxl library and Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
from openpyxl import load_workbook
from tkinter import filedialog
def load_excel_file():
global workbook
filepath = filedialog.askopenfilename(filetypes=[("Excel Files", "*.xlsx")])
if filepath:
workbook = load_workbook(filepath)
sheet_names = workbook.sheetnames
sheet_selector['values'] = sheet_names
sheet_selector.set(sheet_names[0])
display_sheet_data()
def display_sheet_data():
sheet_name = sheet_selector.get()
if sheet_name and workbook:
sheet = workbook[sheet_name]
data_display.delete("1.0", tk.END)
for row in sheet.iter_rows(values_only=True):
row_text = "\t".join(str(cell) for cell in row) + "\n"
data_display.insert(tk.END, row_text)
root = tk.Tk()
root.title("Excel Data Viewer")
file_frame = ttk.LabelFrame(root, text="File")
file_frame.pack(fill=tk.BOTH, expand=True, padx=10, pady=5)
load_button = ttk.Button(file_frame, text="Load Excel File", command=load_excel_file)
load_button.pack(side=tk.LEFT, padx=5)
sheet_selector = ttk.Combobox(file_frame, state="readonly")
sheet_selector.pack(side=tk.LEFT, padx=5)
sheet_selector.bind("<>", lambda event: display_sheet_data())
data_frame = ttk.LabelFrame(root, text="Data")
data_frame.pack(fill=tk.BOTH, expand=True, padx=10, pady=5)
data_display = tk.Text(data_frame, wrap=tk.NONE)
data_display.pack(fill=tk.BOTH, expand=True, padx=5, pady=5)
workbook = None
root.mainloop()
Explanation:
In the exercise above -
- Import the required libraries, including tkinter, ttk, openpyxl, and filedialog.
- Create a function "load_excel_file()" to open an Excel file using the "filedialog.askopenfilename" method and load the selected workbook into the workbook variable.
- Create a function "display_sheet_data()" to display the data from the selected sheet. It clears the existing data in the text widget and iterates through the rows of the selected sheet. It displays the data in tabular format.
- Create the main Tkinter window, add a "Load Excel File" button, a sheet selector dropdown, and a text widget to display the data.
- When the "Load Excel File" button is clicked, the program opens the file dialog to select an "Excel" file.
- The program displays data from the selected sheet in the text widget when it is selected from the dropdown.
Output:
Flowchart:
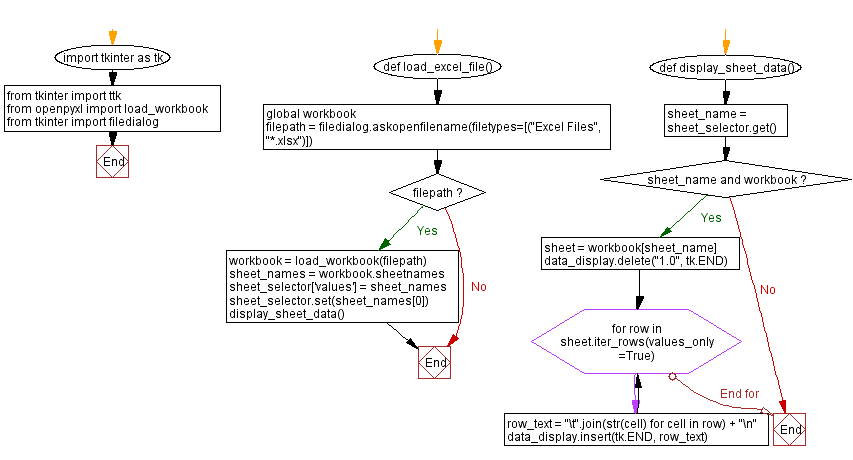
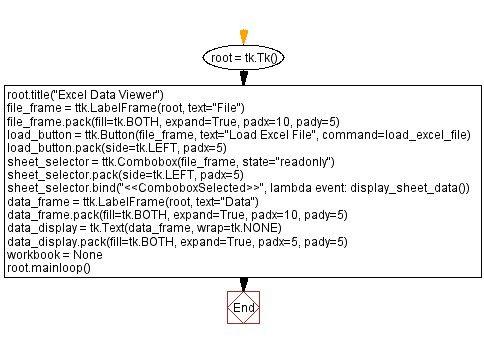
Python Code Editor:
Previous: Python Tkinter Calendar App.
Next: Python Tkinter password manager.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics