Python Tkinter password manager
Python Tkinter File Operations and Integration: Exercise-9 with Solution
Write a Python program that implements a password manager using Tkinter. Allow users to create, save, and retrieve passwords stored in a secure text file.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
# Function to save the password to a text file
def save_password():
website = website_entry.get()
username = username_entry.get()
password = password_entry.get()
# Check if any field is empty
if not website or not username or not password:
messagebox.showerror("Error", "Please fill in all fields.")
return
# Save the password to a text file (not secure)
with open("passwords.txt", "a") as file:
file.write(f"Website: {website}\n")
file.write(f"Username: {username}\n")
file.write(f"Password: {password}\n\n")
# Clear the input fields
website_entry.delete(0, tk.END)
username_entry.delete(0, tk.END)
password_entry.delete(0, tk.END)
# Function to retrieve passwords from the text file
def retrieve_passwords():
passwords = ""
try:
with open("passwords.txt", "r") as file:
passwords = file.read()
except FileNotFoundError:
passwords = "Password file not found."
# Display the passwords in a message box
messagebox.showinfo("Stored Passwords", passwords)
# Create the main window
root = tk.Tk()
root.title("Password Manager")
# Create and pack labels and entry widgets
website_label = tk.Label(root, text="Website:")
website_label.pack()
website_entry = tk.Entry(root)
website_entry.pack()
username_label = tk.Label(root, text="Username:")
username_label.pack()
username_entry = tk.Entry(root)
username_entry.pack()
password_label = tk.Label(root, text="Password:")
password_label.pack()
password_entry = tk.Entry(root, show="*")
password_entry.pack()
# Create and pack buttons
save_button = tk.Button(root, text="Save Password", command=save_password)
save_button.pack()
retrieve_button = tk.Button(root, text="Retrieve Passwords", command=retrieve_passwords)
retrieve_button.pack()
# Run the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the necessary modules.
- Define two functions:
- save_password(): This function is called when the "Save Password" button is clicked. It retrieves the user's website, username, and password and check if any field is empty, and then appends this information to a text file named "passwords.txt." It also clears the input fields.
- retrieve_passwords(): This function is called when the "Retrieve Passwords" button is clicked. It reads the "passwords.txt" file and displays them in a message box.
- Create the main Tkinter window, set its title to "Password Manager," and define labels and entry widgets for website, username, and password input.
- Create buttons for storing and retrieving passwords. These buttons are associated with their respective functions.
- With root.mainloop(), we start the Tkinter event loop, which keeps the GUI running and updates the time.
Output:
Flowchart:
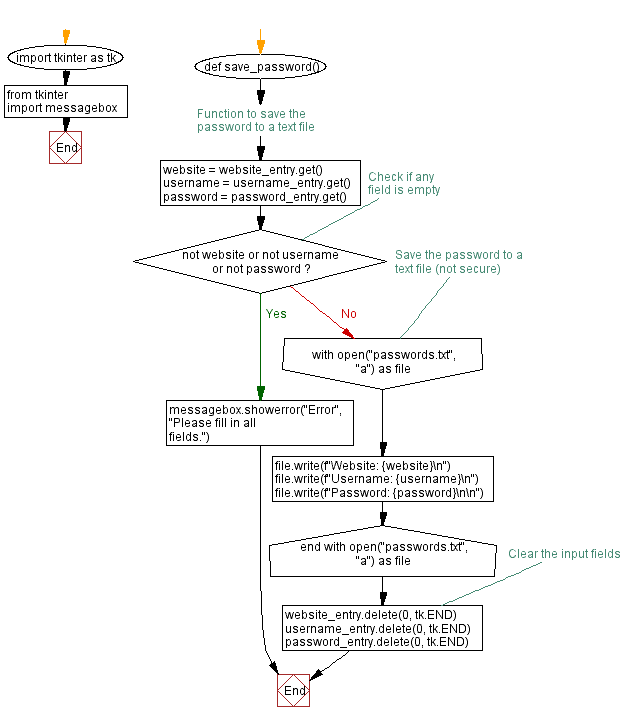
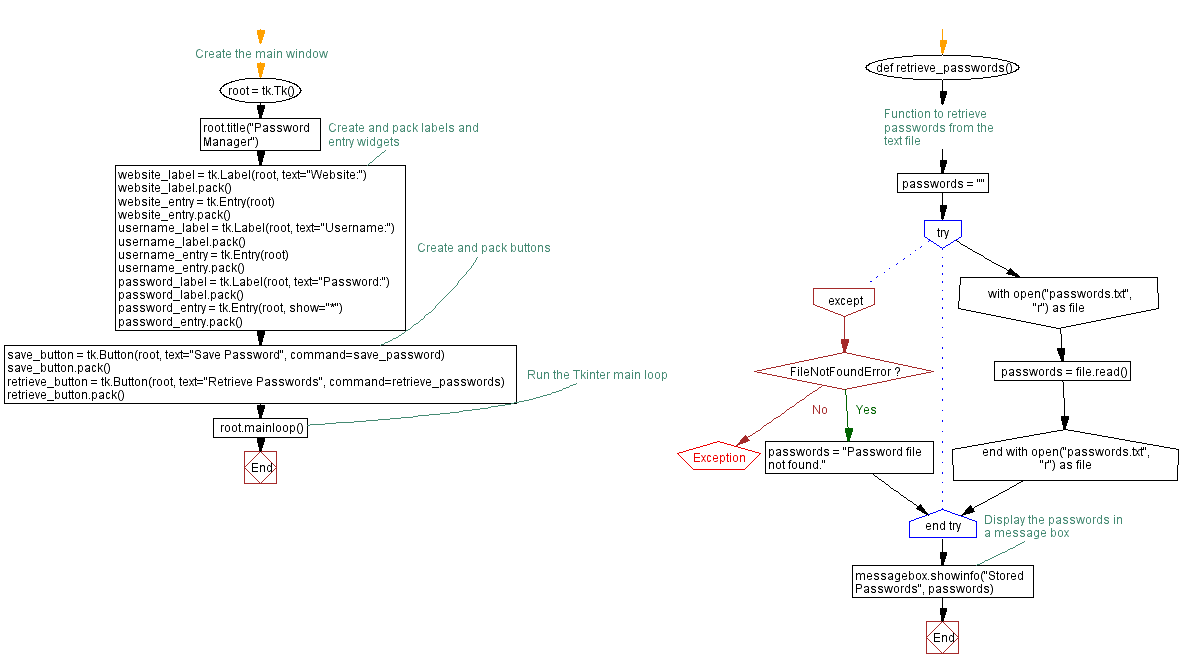
Python Code Editor:
Previous: Python Tkinter Calendar App.
Next: Python web browser with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics