Create a vertical label layout in Python Tkinter
Python tkinter layout management: Exercise-1 with Solution
Write a Python program to create a Tkinter window with three labels arranged vertically using the Pack geometry manager.
Sample Solution:
Python Code:
import tkinter as tk
# Create the main Tkinter window
parent = tk.Tk()
parent.title("Vertical Labels with Pack")
# Create three label widgets
label1 = tk.Label(parent, text="Python Exercises", padx=10, pady=5)
label2 = tk.Label(parent, text="Java Exercises", padx=10, pady=5)
label3 = tk.Label(parent, text="C++ Exercises", padx=10, pady=5)
# Use the Pack geometry manager to arrange labels vertically
label1.pack(side="top")
label2.pack(side="top")
label3.pack(side="top")
# Run the Tkinter main loop
parent.mainloop()
Explanation:
In the exercise above -
- import the tkinter module as tk.
- Create the main Tkinter window using tk.Tk() and set its title to "Vertical Labels with Pack."
- Create three label widgets (label1, label2, and label3) with different text labels and some padding (using padx and pady) for spacing.
- Use the pack() method to arrange labels vertically. By specifying side="top" for each label, they will be stacked below the other in the order they were packed.
- Finally, start the Tkinter main loop with parent.mainloop(), which keeps the GUI application running and displays the vertically arranged labels in the window.
Sample Output:
Flowchart:
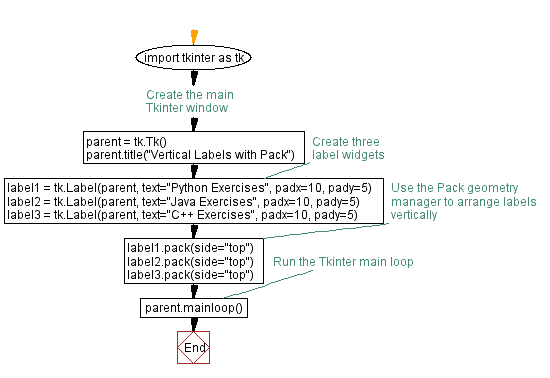
Python Code Editor:
Previous: Python tkinter layout management Home.
Next: Organize widgets with Python Tkinter's grid manager.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics