Create an employee list application with Python Tkinter
Python tkinter layout management: Exercise-6 with Solution
Write a Python program that builds an employee list application with a Listbox widget to display names. Use the Pack geometry manager to align the Listbox and buttons.
Sample Solution:
Python Code:
import tkinter as tk
# Function to add a new contact to the Listbox
def add_contact():
name = name_entry.get()
if name:
contacts_listbox.insert(tk.END, name)
name_entry.delete(0, tk.END)
# Function to delete the selected contact from the Listbox
def delete_contact():
selected_index = contacts_listbox.curselection()
if selected_index:
contacts_listbox.delete(selected_index)
# Create the main window
root = tk.Tk()
root.title("Employee List Application")
# Create a Listbox to display contacts
contacts_listbox = tk.Listbox(root, selectmode=tk.SINGLE)
contacts_listbox.pack(padx=10, pady=10, fill=tk.BOTH, expand=True)
# Create an Entry widget to input new contacts
name_entry = tk.Entry(root)
name_entry.pack(pady=5)
# Create "Add" and "Delete" buttons
add_button = tk.Button(root, text="Add", command=add_contact)
add_button.pack(side=tk.LEFT, padx=5)
delete_button = tk.Button(root, text="Delete", command=delete_contact)
delete_button.pack(side=tk.LEFT, padx=5)
# Start the Tkinter event loop
root.mainloop()
Explanation:
In the exercise above -
- Import the tkinter library.
- The "add_contact()" function adds a new contact (name) to the Listbox when the "Add" button is clicked. It retrieves the name from the Entry widget and inserts it into the Listbox.
- The "delete_contact()" function deletes the selected contact from the Listbox when the "Delete" button is clicked. It first gets the index of the selected item and deletes it from the Listbox.
- Create the main window and set its title.
- A Listbox is created to display the list of names of employees, and it is configured to allow single-item selection.
- An 'Entry' widget is used for entering new contact names.
- "Add" and "Delete" buttons are created and packed to the left side of the window.
- The pack method is used to arrange the widgets within the main window using the Pack geometry manager. Listboxes expand and fill available space when the fill and expand parameters are set to tk.BOTH and True, respectively.
- With root.mainloop(), the Tkinter GUI runs and responds to user interactions.
Sample Output:
Flowchart:
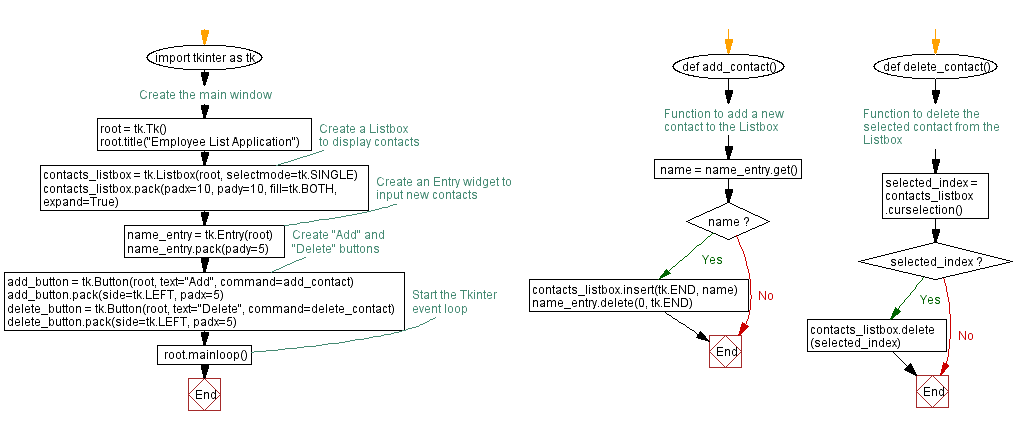
Python Code Editor:
Previous: Create a Python login form with Tkinter's grid manager.
Next: Create a chat application interface with Python Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics