C Exercises: Delete an element at desired position from an array
15. Delete Element from Array
Write a program in C to delete an element at a desired position from an array.
Visual Presentation:
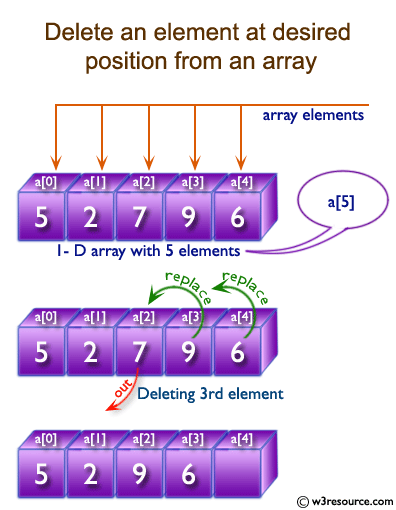
Sample Solution:
C Code:
#include <stdio.h>
void main() {
int arr1[50], i, pos, n;
// Prompt user for input
printf("\n\nDelete an element at the desired position from an array:\n");
printf("---------------------------------------------------------\n");
printf("Input the size of the array : ");
scanf("%d", &n);
// Input values for the array
printf("Input %d elements in the array in ascending order:\n", n);
for (i = 0; i < n; i++) {
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Input the position to delete
printf("\nInput the position where to delete: ");
scanf("%d", &pos);
// Locate the position in the array
i = 0;
while (i != pos - 1)
i++;
// Shift elements to the left to replace the deleted element
while (i < n) {
arr1[i] = arr1[i + 1];
i++;
}
n--;
// Display the new list after deletion
printf("\nThe new list is : ");
for (i = 0; i < n; i++) {
printf(" %d", arr1[i]);
}
printf("\n\n");
}
Sample Output:
Delete an element at desired position from an array : --------------------------------------------------------- Input the size of array : 5 Input 5 elements in the array in ascending order: element - 0 : 1 element - 1 : 2 element - 2 : 3 element - 3 : 4 element - 4 : 5 Input the position where to delete: 3 The new list is : 1 2 4 5
Flowchart:
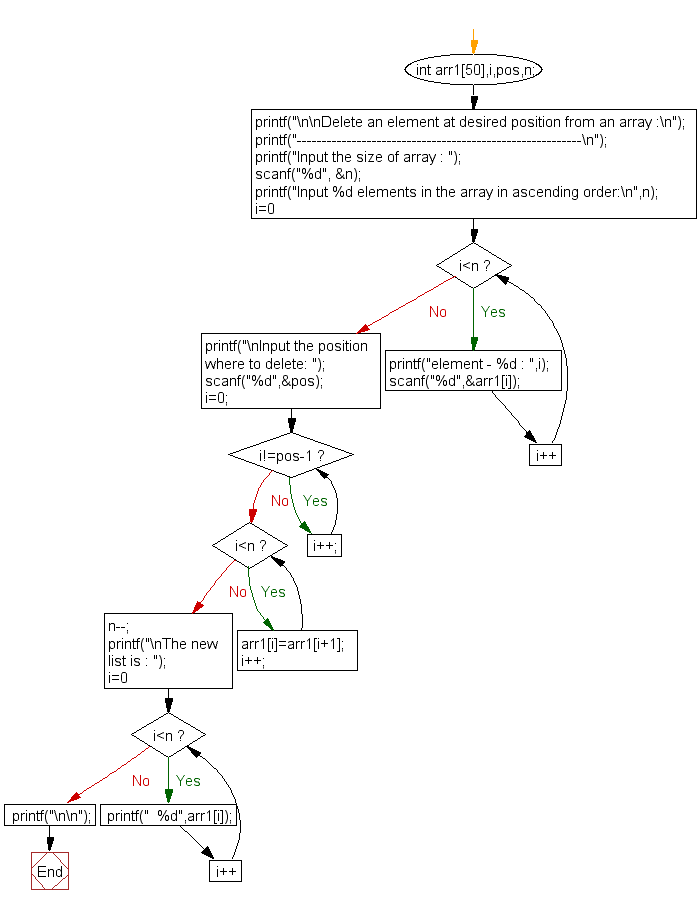
For more Practice: Solve these Related Problems:
- Write a C program to delete an element from an array at a given position using pointer arithmetic.
- Write a C program to delete an element from an array and then shift the remaining elements to fill the gap.
- Write a C program to delete multiple elements from an array based on user-specified positions.
- Write a C program to delete an element from an array and then display the updated array in reverse order.
C Programming Code Editor:
Previous: Write a program in C to insert New value in the array (unsorted list ).
Next: Write a program in C to find the second largest element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.