C Exercises: Find the second smallest element in an array
17. Second Smallest Element
Write a program in C to find the second smallest element in an array.
The task is to develop a C program that identifies the second smallest element in an array. It prompts the user for the array size and elements, ensuring each element is less than 9999, and then determines and displays the second smallest element in the array.
Visual Presentation:
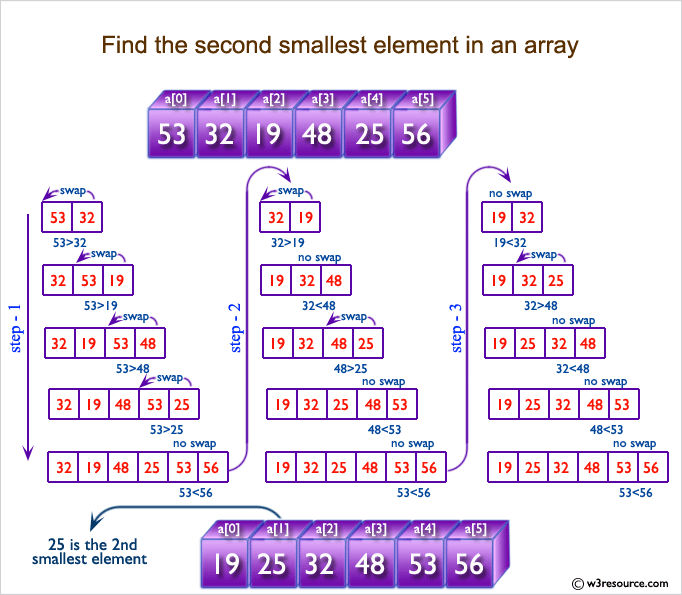
Sample Solution:
C Code:
#include <stdio.h>
void main() {
int arr1[50], n, i, j = 0, sml, sml2nd;
// Prompt user for input
printf("\n\nFind the second smallest element in an array :\n");
printf("--------------------------------------------------\n");
printf("Input the size of the array : ");
scanf("%d", &n);
// Input values for the array
printf("Input %d elements in the array (value must be <9999) :\n", n);
for (i = 0; i < n; i++) {
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Find the location of the smallest element in the array
sml = arr1[0];
for (i = 0; i < n; i++) {
if (sml > arr1[i]) {
sml = arr1[i];
j = i;
}
}
// Ignore the smallest element and find the second smallest element in the array
sml2nd = 99999;
for (i = 0; i < n; i++) {
if (i == j) {
i++; // Ignore the smallest element
i--;
} else {
if (sml2nd > arr1[i]) {
sml2nd = arr1[i];
}
}
}
// Display the second smallest element
printf("The Second smallest element in the array is : %d \n\n", sml2nd);
}
Sample Output:
Find the second smallest element in an array : -------------------------------------------------- Input the size of array : 5 Input 5 elements in the array (value must be <9999) : element - 0 : 0 element - 1 : 9 element - 2 : 4 element - 3 : 6 element - 4 : 5 The Second smallest element in the array is : 4
Flowchart:
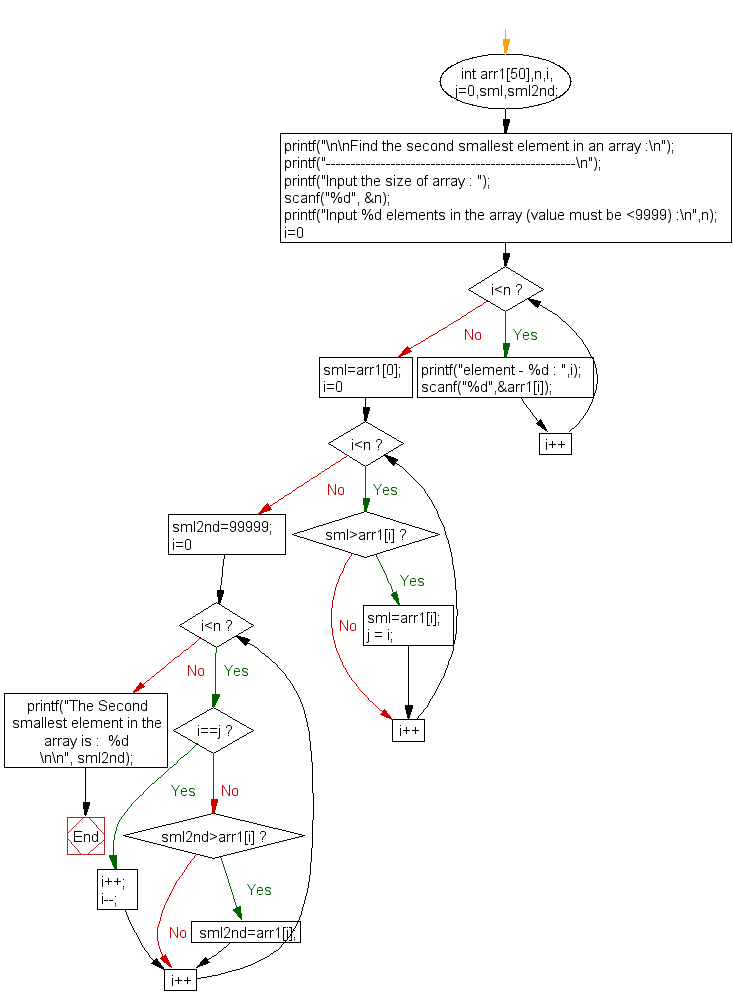
For more Practice: Solve these Related Problems:
- Write a C program to find the second smallest element in an array without sorting.
- Write a C program to identify the second smallest element using two passes through the array.
- Write a C program to find the second smallest element and then display the array with it highlighted.
- Write a C program to determine the second smallest element using recursion.
C Programming Code Editor:
Previous: Write a program in C to find the second largest element in an array.
Next: Write a program in C for a 2D array of size 3x3 and print the matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.