C Exercises: Find the missing number from a given array
36. Find Missing Number in Array
Write a program in C to find the missing number in a given array. There are no duplicates in the list.
To solve the problem of finding the missing number in a given array of unique integers, you can implement a C program that utilizes mathematical formulas. Calculate the expected sum of the sequence up to the length of the array, then subtract the sum of the array's elements to find the missing number. This approach efficiently identifies the missing number without requiring additional space or complex algorithms.
Visual Presentation:
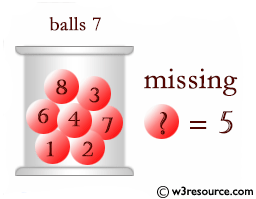
Sample Solution:
C Code:
#include <stdio.h>
// Function to find the missing number in an array
int pickMissNumber(int *arr1, int ar_size) {
int i, sum = 0, n = ar_size + 1;
// Calculating the sum of all elements in the array
for (i = 0; i < ar_size; i++) {
sum = sum + arr1[i];
}
// Calculating the missing number using the sum of the first 'n' natural numbers formula
return (n * (n + 1)) / 2 - sum;
}
// Main function
int main() {
int i;
int arr1[] = {1, 3, 4, 2, 5, 6, 9, 8};
int ctr = sizeof(arr1) / sizeof(arr1[0]);
// Displaying the given array
printf("The given array is : ");
for (i = 0; i < ctr; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
// Finding and displaying the missing number
printf("The missing number is : %d \n", pickMissNumber(arr1, ctr));
return 0;
}
Sample Output:
The given array is : 1 3 4 2 5 6 9 8 The missing number is : 7
Flowchart :
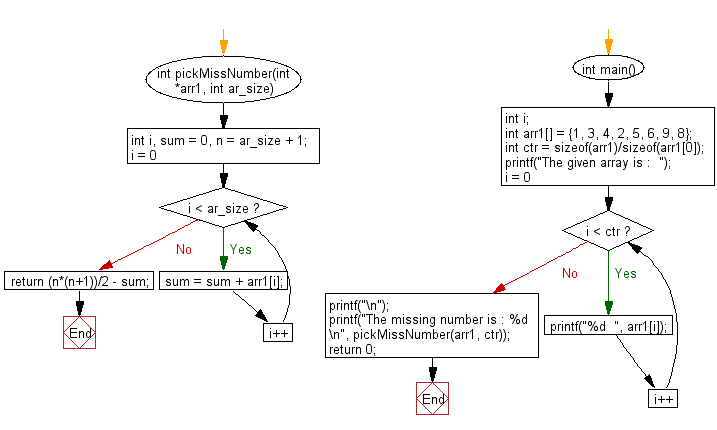
For more Practice: Solve these Related Problems:
- Write a C program to find the missing number in an array using the sum formula.
- Write a C program to identify the missing number in an array by sorting and comparing consecutive elements.
- Write a C program to find the missing number using XOR operation on all elements.
- Write a C program to find the missing number in an unsorted array by using a hash table.
C Programming Code Editor:
Previous: Write a program in C to find the largest sum of contiguous subarray of an array.
Next: Write a program in C to find the pivot element of a sorted and rotated array using binary search
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.