C Exercises: Find the next greater elements in a given unsorted array
43. Next Greatest Elements in Unsorted Array
Write a program in C to print the next greatest elements in a given unsorted array. Elements for which no superior element exists, consider the next greatest element as -1.
The task is to find the next greatest element for each element in an unsorted array. For each element, the program finds the nearest greater element on its right; if none exists, it assigns -1.
Visual Presentation:
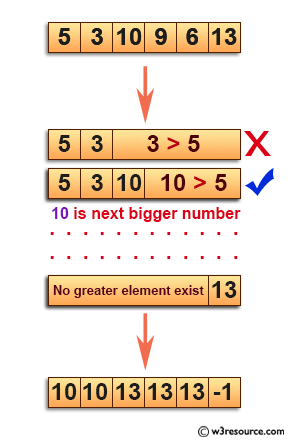
Sample Solution:
C Code:
#include <stdio.h>
// Function to find the next larger element for each element in the array
void findNxtLrgElem(int *arr1, int arr1_size) {
int nxtBgElem, i, j;
for(i = 0; i < arr1_size; i++) {
// Find the next bigger element for arr1[i]
for (j = i + 1, nxtBgElem = -1; j < arr1_size; j++) {
if (arr1[i] < arr1[j]) {
nxtBgElem = arr1[j];
break;
}
}
printf("Next bigger element of %d in the array is: %d\n", arr1[i], nxtBgElem);
}
}
// Function to form an array with next bigger elements
void formBigElemArray(int *arr1, int arr1_size) {
int nxtBgElem, i, j;
for(i = 0; i < arr1_size; i++) {
// Find the next bigger element for arr1[i]
for (j = i + 1, nxtBgElem = -1; j < arr1_size; j++) {
if (arr1[i] < arr1[j]) {
nxtBgElem = arr1[j];
break;
}
}
printf("%d ", nxtBgElem);
}
}
int main() {
int i, arr1[] = {5, 3, 10, 9, 6, 13};
int ctr = sizeof(arr1) / sizeof(arr1[0]);
// Print the original array
printf("The given array is : ");
for(i = 0; i < ctr; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
// Find and print the next bigger elements for each element in the array
printf("\nNext Bigger Elements are:\n");
findNxtLrgElem(arr1, ctr);
// Form and print an array with next bigger elements
printf("\nNext Bigger Elements Array:\n");
formBigElemArray(arr1, ctr);
return 0;
}
Sample Output:
The given array is : 5 3 10 9 6 13 Next Bigger Elements are: Next bigger element of 5 in the array is: 10 Next bigger element of 3 in the array is: 10 Next bigger element of 10 in the array is: 13 Next bigger element of 9 in the array is: 13 Next bigger element of 6 in the array is: 13 Next bigger element of 13 in the array is: -1 Next Bigger Elements Array: 10 10 13 13 13 -1
Flowchart:
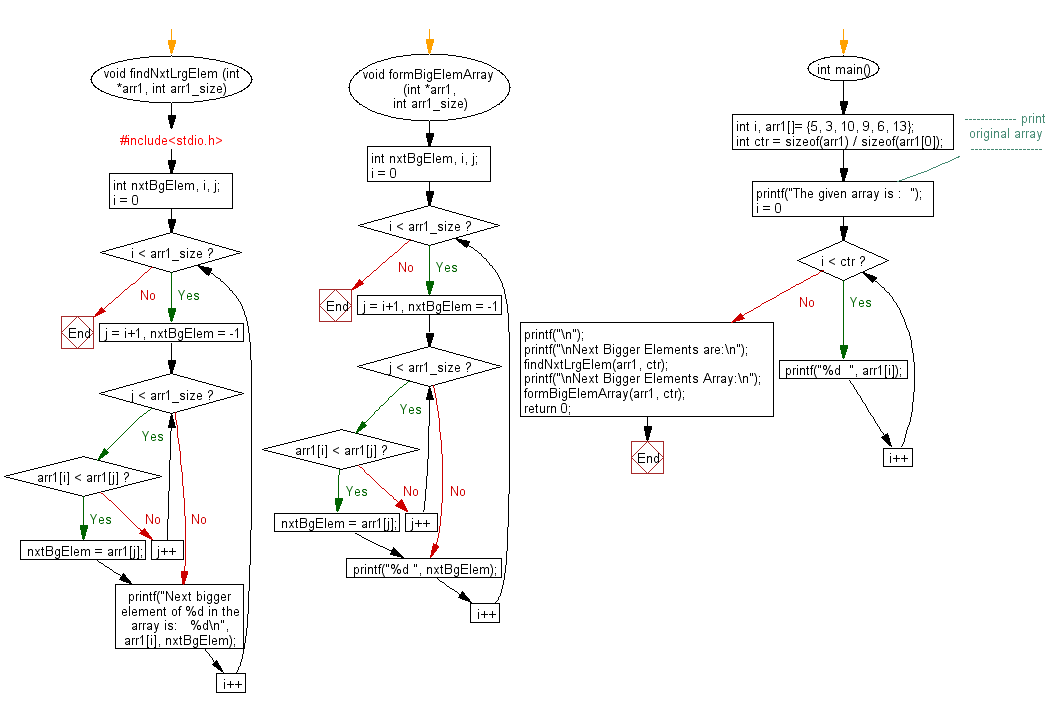
For more Practice: Solve these Related Problems:
- Write a C program to print the next greater element for each element in an unsorted array using a stack.
- Write a C program to find the next greater element for each array element without using extra space.
- Write a C program to display the next greater element for every element and then count the total such elements.
- Write a C program to find and print the next greater elements recursively.
C Programming Code Editor:
Previous: Write a program in C to find the smallest missing element from a sorted array.
Next: Write a program in C to find the two repeating elements in a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.