C Exercises: Find the row with maximum number of 1s
60. Row with Maximum 1s in 2D Array
Write a program in C to find the row with the maximum number of 1s.
Expected Output :
The given 2D array is :
0 1 0 1 1
1 1 1 1 1
1 0 0 1 0
0 0 0 0 0
1 0 0 0 1
The index of row with maximum 1s is: 1
To find the row with the maximum number of 1s in a 2D array, the program iterates through each row, counting the number of 1s in each. By keeping track of the maximum count and the corresponding row index, the program determines which row contains the most 1s. This approach ensures an efficient comparison across all rows to identify the desired row index.
Visual Presentation: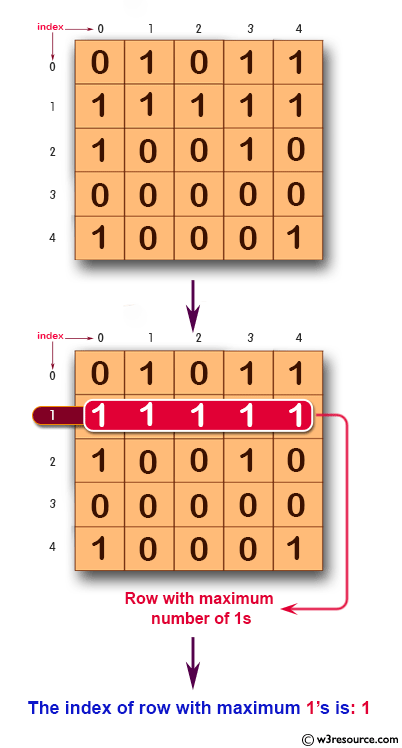
Sample Solution:
C Code:
#include <stdio.h>
#define R 5
#define C 5
// Function to find the index of the first occurrence of 1 in an array
int getFirstOccur(int arr1[], int l, int h) {
if (h >= l) {
int mid = l + (h - l) / 2;
// Checking conditions to find the first occurrence of 1
if ((mid == 0 || arr1[mid - 1] == 0) && arr1[mid] == 1)
return mid;
else if (arr1[mid] == 0)
return getFirstOccur(arr1, (mid + 1), h);
else
return getFirstOccur(arr1, l, (mid - 1));
}
return -1;
}
// Function to find the row with the maximum number of 1s
int findRowMaxOne(int arr2d[R][C]) {
int max_row_index = 0, max = -1;
int i, index;
// Loop through each row to find the row with maximum 1s
for (i = 0; i < R; i++) {
index = getFirstOccur(arr2d[i], 0, C - 1); // Get index of first occurrence of 1
// Update max_row_index if a row with more 1s is found
if (index != -1 && C - index > max) {
max = C - index;
max_row_index = i;
}
}
return max_row_index;
}
int main() {
int arr2d[R][C] = {
{0, 1, 0, 1, 1},
{1, 1, 1, 1, 1},
{1, 0, 0, 1, 0},
{0, 0, 0, 0, 0},
{1, 0, 0, 0, 1}
};
int i, j;
//------------- print original 2D array ------------------
printf("The given 2D array is : \n");
for(i = 0; i < R; i++) {
for(j = 0; j < C; j++) {
printf("%d ", arr2d[i][j]);
}
printf("\n");
}
//------------------------------------------------------
printf("The index of row with maximum 1s is: %d ", findRowMaxOne(arr2d));
return 0;
}
Output:
The given 2D array is : 0 1 0 1 1 1 1 1 1 1 1 0 0 1 0 0 0 0 0 0 1 0 0 0 1 The index of row with maximum 1s is: 1
For more Practice: Solve these Related Problems:
- Write a C program to find the row with the maximum number of 1s in a binary matrix using efficient row scanning.
- Write a C program to count the number of 1s in each row of a 2D array and then display the row with the highest count.
- Write a C program to find the row with maximum 1s using pointer arithmetic on a dynamically allocated 2D array.
- Write a C program to identify the row with the maximum 1s and then compute the sum of that row’s elements.
C Programming Code Editor:
Previous: Write a program in C to return the counting sort on an array.
Next: Write a program in C to find maximum product subarray in a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.