C Exercises: Count the number of inversion in a given array
66. Count Inversions in Array
Write a program in C to count the number of inversions in a given array.
Expected Output :
The given array is : 1 9 6 4 5
The inversions are: (9, 6) (9, 4) (9, 5) (6, 4) (6, 5)
The number of inversion can be formed from the array is: 5
The problem involves writing a C program to count the number of inversions in a given array. An inversion is a pair of elements where the first element is greater than the second element and appears before it in the array. The program should identify and count all such pairs, then display both the inversions and their total count.
Visual Presentation:
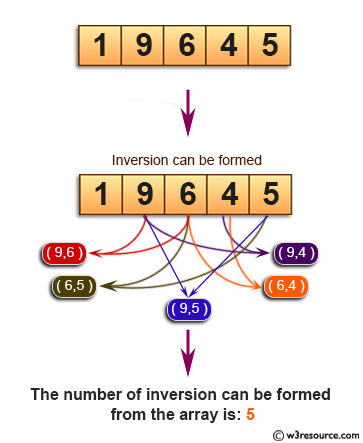
Sample Solution:
C Code:
#include <stdio.h>
// Function to count the number of inversions in the array
int inv_count(int arr1[], int n) {
int inversionCtr = 0;
printf("The inversions are: ");
// Loop through each pair of elements to find inversions
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
// If a pair forms an inversion, print it and increment the counter
if (arr1[i] > arr1[j]) {
printf("(%d, %d) ", arr1[i], arr1[j]);
inversionCtr++;
}
}
}
return inversionCtr; // Return the total count of inversions
}
int main() {
int arr1[] = { 1, 9, 6, 4, 5 };
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
// Print the original array
printf("The given array is : ");
for(i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
// Count and display the number of inversions in the array
printf("\nThe number of inversions that can be formed from the array is: %d", inv_count(arr1, n));
return 0;
}
Sample Output:
The given array is : 1 9 6 4 5 The inversions are: (9, 6) (9, 4) (9, 5) (6, 4) (6, 5) The number of inversion can be formed from the array is: 5
Flowchart:/p>
For more Practice: Solve these Related Problems:
- Write a C program to count inversions in an array using a modified merge sort algorithm.
- Write a C program to count inversions recursively and then output all inversion pairs.
- Write a C program to count the number of inversions in an array using brute-force nested loops.
- Write a C program to compute the inversion count and then compare it with the sorted order of the array.
C Programming Code Editor:
Previous: Write a program in C to find the product of an array such that product is equal to the product of all the elements of arr[] except arr[i].
Next: Write a program in C to search an element in a row wise and column wise sorted matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.