C Program: Calculate Sum of positive integers using Do-While Loop
2. Sum of Positive Integers Until 0
Write a C program that prompts the user to input a series of integers until the user stops by entering 0 using a do-while loop. Calculate and print the sum of all positive integers entered.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int num, sum = 0; // Declare variables to store the entered number and the sum
// Prompt the user to input integers until they enter 0
do {
printf("Input an integer (enter 0 to stop): ");
scanf("%d", &num); // Read the entered number
if (num > 0) {
sum += num; // Add the positive number to the sum
} else if (num < 0) {
printf("Negative integers are not considered. Ignored.\n");
}
} while (num != 0); // Continue the loop until the user enters 0
// Print the sum of positive integers
printf("Sum of positive integers: %d\n", sum);
return 0; // Indicate successful program execution
}
Sample Output:
Input an integer (enter 0 to stop): 4 Input an integer (enter 0 to stop): 3 Input an integer (enter 0 to stop): 2 Input an integer (enter 0 to stop): -7 Negative integers are not considered. Ignored. Input an integer (enter 0 to stop): 5 Input an integer (enter 0 to stop): 0 Sum of positive integers: 14
Explanation:
Here is the break down of the above code step by step:
- #include <stdio.h>: This line includes the standard input-output library, which is necessary for using functions like "printf()" and "scanf()".
- int main() {: This line marks the beginning of the "main()" function, which is the entry point of every C program.
- int num, sum = 0;: This line declares two integer variables, 'num' to store the entered number, and 'sum' to store the sum of positive integers. 'sum' is initialized to 0.
- // Prompt the user to input integers until they enter 0: Comment indicating the intention of the following code block.
- do {: Marks the beginning of a do-while loop. The code inside the curly braces will be executed at least once before checking the loop condition.
- printf("Input an integer (enter 0 to stop): ");: Displays a prompt asking the user to input an integer, with an additional instruction to enter 0 to stop.
- scanf("%d", &num);: Reads the entered integer from the user and stores it in the variable num.
- if (num > 0) {: Checks if the entered number is positive.
- sum += num;: If the number is positive, it is added to the sum variable.
- } else if (num < 0) {: If the number is negative, this block is executed.
- printf("Negative integers are not considered. Ignored.\n");: Displays a message indicating that negative integers are not considered, and they are ignored.
- } while (num != 0);: Specifies the loop condition. The loop continues as long as the user does not enter 0.
- // Print the sum of positive integers: Comment indicating the intention of the following code block.
- printf("Sum of positive integers: %d\n", sum);: Prints the sum of positive integers calculated during the loop.
- return 0;: Indicates that the program executed successfully. The 0 here is typically used to indicate successful execution.
- }: Marks the end of the main function.
Flowchart:
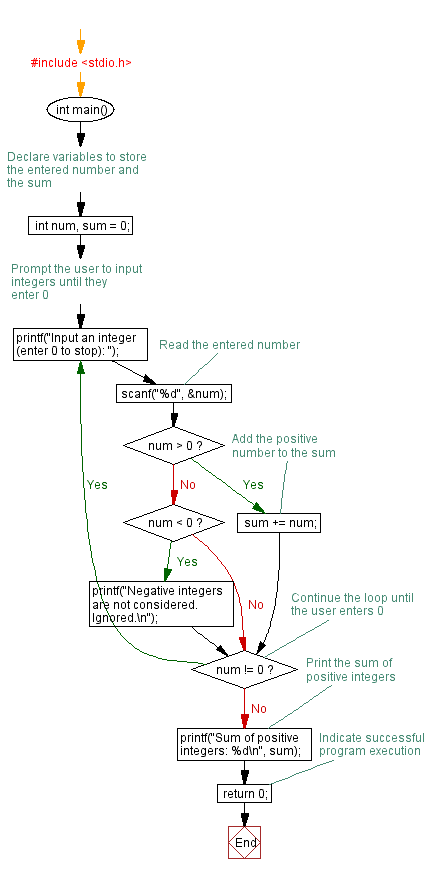
For more Practice: Solve these Related Problems:
- Write a C program to accept numbers until 0 is entered and then print the sum of even numbers only.
- Write a C program to accept numbers until 0 is entered and count how many numbers were entered before 0.
- Write a C program to accept numbers until 0 is entered and display the largest and smallest numbers entered.
- Write a C program to accept numbers until 0 is entered and calculate the sum of the absolute values of all numbers.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: C Program to Print numbers from 1 to 10 and 10 to 1 using Do-While Loop.
Next: Calculate Sum of even and odd numbers (1-50) with Do-While Loops.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.