C Exercises: Check if a given integer is prime using an inline function
8. Prime Check Inline Variants
Write a C program to check if a given integer is prime using an inline function.
Sample Solution:
C Code:
#include <stdio.h>
// inline function to check if a given integer is prime
inline int is_Prime(int n) {
if (n <= 1) {
return 0;
}
for (int i = 2; i * i <= n; i++) {
if (n % i == 0) {
return 0;
}
}
return 1;
}
int main() {
int num;
printf("Input a positive integer: ");
scanf("%d", & num);
if (is_Prime(num)) {
printf("%d is a prime number.\n", num);
} else {
printf("%d is not a prime number.\n", num);
}
return 0;
}
Sample Output:
Input a positive integer: 13 13 is a prime number. Input a positive integer: 18 18 is not a prime number.
Explanation:
The is_prime() function takes an integer as an argument and returns 1 if it is prime and 0 otherwise. It works by iterating through all numbers from 2 up to the square root of the given number. It checks if the number is divisible by any of those numbers. If it is, it's not prime and the function returns 0. If it's not divisible by any of those numbers, it's prime and the function returns 1.
The main() function asks the user to input a positive integer, then uses the isPrime() inline function to check if the number is prime or not, and prints the result.
Flowchart:
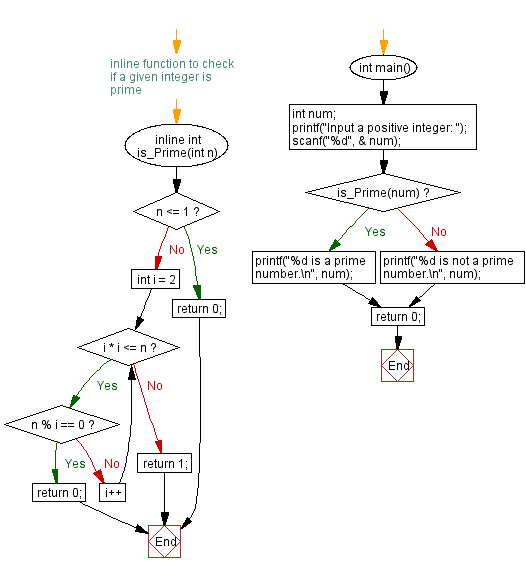
For more Practice: Solve these Related Problems:
- Write a C program to check if an integer is prime using an optimized inline function that tests divisibility only up to the square root.
- Write a C program to verify the primality of a number using recursion within an inline function.
- Write a C program to implement an inline function that returns a boolean indicating prime status for unsigned integers.
- Write a C program to list all prime numbers within a range by invoking an inline prime-check function for each candidate.
C Programming Code Editor:
Previous: Count the number of vowels in a given string using an inline function.
Next: Check if a given integer is even or odd using an inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.