C Exercises: Convert a Singly Linked list into a array
12. Linked List to Array Variants
Write a C program that converts a singly linked list into an array and returns it.
Sample Solution:
C Code:
#include<stdio.h>
#include <stdlib.h>
#include <string.h>
// Structure for a node in a singly linked list
struct node
{
int num; // Data of the node
struct node *nextptr; // Address of the next node
} *stnode; // Pointer to the start of the linked list
// Function prototypes
void createNodeList(int n);
void To_Array(struct node *head, int *array);
int main()
{
int n;
printf("\nLinked List: Convert a Singly Linked list into an array:\n");
printf("-------------------------------------------------------------\n");
printf("Input the number of nodes: ");
scanf("%d", &n);
// Creating a singly linked list
createNodeList(n);
// Converting the linked list to an array
printf("\nReturn data entered in the list as an array:\n");
int arr[n];
To_Array(stnode, arr);
// Displaying the elements of the array
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
return 0;
}
// Function to create a singly linked list with 'n' nodes
void createNodeList(int n)
{
struct node *fnNode, *tmp;
int num, i;
// Allocating memory for the first node
stnode = (struct node *)malloc(sizeof(struct node));
if(stnode == NULL) // Check if memory allocation failed
{
printf(" Memory can not be allocated.");
}
else
{
// Input data for the first node
printf(" Input data for node 1 : ");
scanf("%d", &num);
stnode->num = num;
stnode->nextptr = NULL;
tmp = stnode;
// Creating 'n' nodes and adding to the linked list
for(i = 2; i <= n; i++)
{
fnNode = (struct node *)malloc(sizeof(struct node));
if(fnNode == NULL)
{
printf(" Memory can not be allocated.");
break;
}
else
{
// Input data for each node
printf(" Input data for node %d : ", i);
scanf(" %d", &num);
fnNode->num = num;
fnNode->nextptr = NULL;
tmp->nextptr = fnNode;
tmp = tmp->nextptr;
}
}
}
}
// Function to convert a singly linked list into an array
void To_Array(struct node *head, int *array) {
struct node *current = head;
int i = 0;
// Traverse the linked list and copy elements to the array
while (current != NULL) {
array[i] = current->num;
current = current->nextptr;
i++;
}
}
Sample Output:
Linked List: Convert a Singly Linked list into an array: ------------------------------------------------------------- Input the number of nodes: 4 Input data for node 1 : 10 Input data for node 2 : 20 Input data for node 3 : 30 Input data for node 4 : 40 Return data entered in the list as an array: 10 20 30 40
Flowchart :
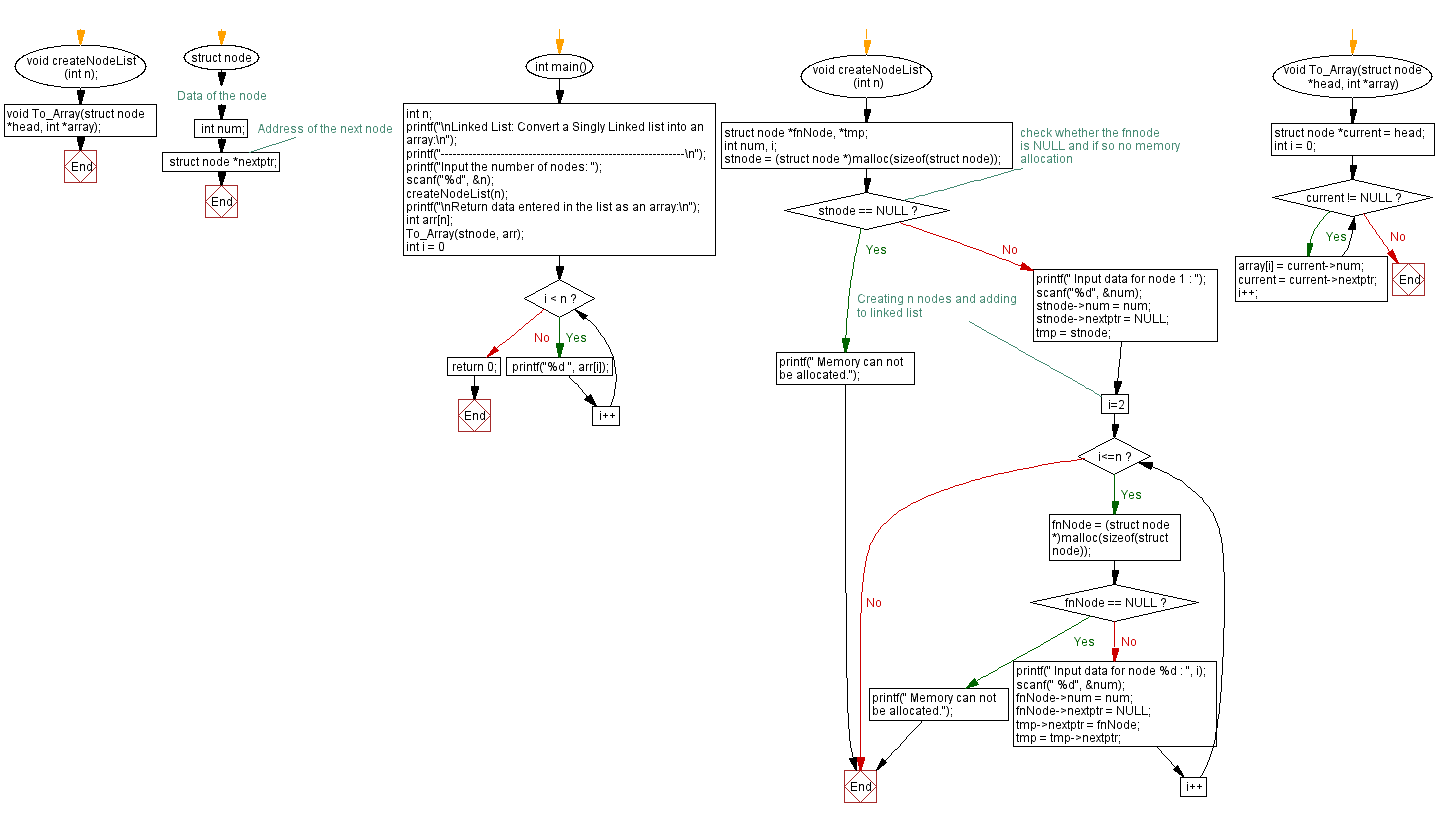
For more Practice: Solve these Related Problems:
- Write a C program to convert a singly linked list into a dynamically allocated array.
- Write a C program to convert a linked list into an array and then sort the array elements.
- Write a C program to convert only the first half of a linked list into an array.
- Write a C program to extract nodes that satisfy a given condition (e.g., prime numbers) into an array.
C Programming Code Editor:
Previous: Convert a Singly Linked list into a string.
Next: Combine two sorted singly linked lists.
What is the difficulty level of this exercise?