C Exercises: Delete all elements greater than x from a linked list
C Singly Linked List : Exercise-37 with Solution
Write a C program to remove all elements from a singly linked list that are greater than a given value x.
Sample Solution:
C Code:
#include<stdio.h>
#include<stdlib.h>
// Structure defining a node in a singly linked list
struct Node {
int data; // Data stored in the node
struct Node* next; // Pointer to the next node
};
// Function to create a new node with given data
struct Node* newNode(int data) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node)); // Allocate memory for a new node
node->data = data; // Assign data to the new node
node->next = NULL; // Initialize the next pointer as NULL
return node; // Return the newly created node
}
// Function to delete all elements greater than x from a linked list
void remove_specific(struct Node** head, int x) {
struct Node* temp = *head; // Initialize a temporary pointer to the head of the list
struct Node* prev = NULL; // Pointer to store the previous node
// Removing nodes with data greater than x from the start of the list
while (temp != NULL && temp->data > x) {
*head = temp->next; // Update the head to the next node
free(temp); // Free the memory of the removed node
temp = *head; // Move temp to the new head
}
// Traverse the remaining list to remove nodes with data greater than x
while (temp != NULL) {
// Traverse until finding a node with data less than or equal to x
while (temp != NULL && temp->data <= x) {
prev = temp; // Store the current node as previous
temp = temp->next; // Move to the next node
}
// If all remaining nodes are less than or equal to x, exit
if (temp == NULL) {
return;
}
// Link the previous node to the next node of temp and free temp node
prev->next = temp->next;
free(temp);
temp = prev->next;
}
}
// Function to print elements of a linked list
void displayList(struct Node* head) {
while (head) {
printf("%d ", head->data); // Print the data of the current node
head = head->next; // Move to the next node
}
printf("\n");
}
// Main function to demonstrate removing elements greater than x from a linked list
int main() {
struct Node* head = newNode(1); // Create the linked list with some initial nodes
head->next = newNode(2);
head->next->next = newNode(3);
head->next->next->next = newNode(4);
head->next->next->next->next = newNode(5);
head->next->next->next->next->next = newNode(6);
head->next->next->next->next->next ->next = newNode(7);
printf("Original singly linked list:\n");
displayList(head); // Display the original list
printf("\nRemove all elements from the said linked list that are greater than 5:\n");
remove_specific(&head, 5); // Remove elements greater than 5 from the list
displayList(head); // Display the modified list after removal
return 0;
}
Sample Output:
Original singly linked list: 1 2 3 4 5 6 7 Remove all elements from the said linked list that are greater than 5: 1 2 3 4 5
Flowchart :
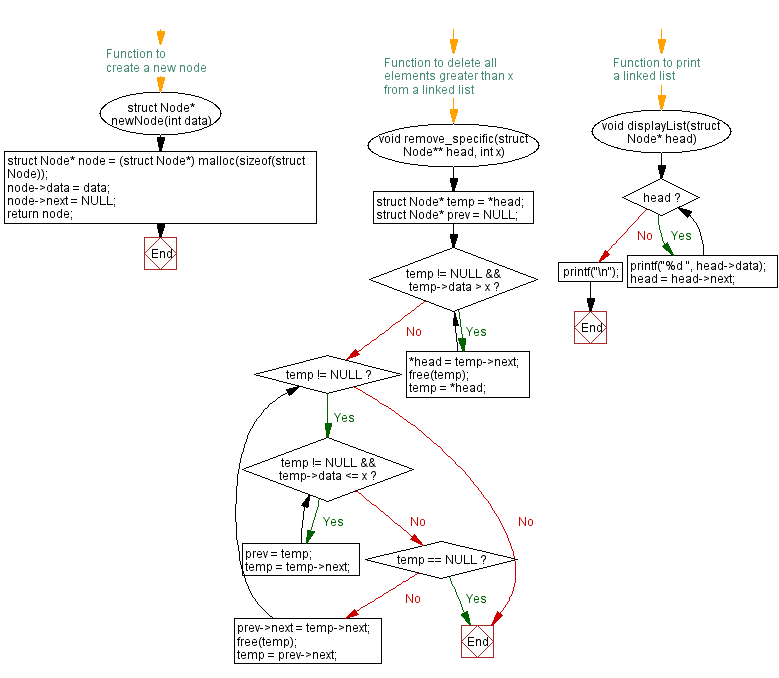
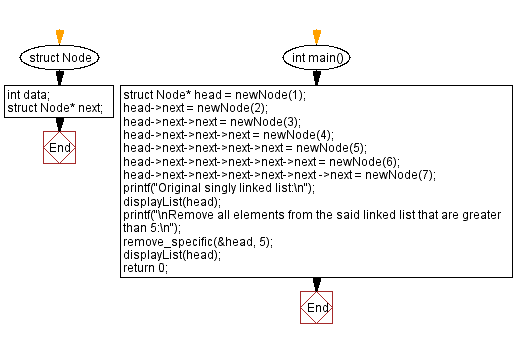
C Programming Code Editor:
Previous: Reverse a singly linked list in blocks of size k.
Next: Pair in a linked list whose sum is equal to a given value.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/linked_list/c-linked_list-exercise-59.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics