C Program structure: Car rental cost calculation
9. Car Structure Management
Design a structure named "Car" to store details like car ID, model, and rental rate per day. Write a C program to input data for three cars, calculate the total rental cost for a specified number of days, and display the results.
Sample Solution:
C Code:
#include <stdio.h>
// Define the structure "Car"
struct Car {
int carID;
char model[50];
float rentalRatePerDay;
};
// Function to calculate the total rental cost for a specified number of days
float calculateRentalCost(struct Car car, int days) {
return car.rentalRatePerDay * days;
}
int main() {
// Declare variables to store details for three cars
struct Car car1, car2, car3;
int rentalDays;
// Input details for the first car
printf("Input details for Car 1:\n");
printf("Car ID: ");
scanf("%d", &car1.carID);
printf("Model: ");
scanf("%s", car1.model); // Assuming models do not contain spaces
printf("Rental Rate per Day: ");
scanf("%f", &car1.rentalRatePerDay);
// Input details for the second car
printf("\nInput details for Car 2:\n");
printf("Car ID: ");
scanf("%d", &car2.carID);
printf("Model: ");
scanf("%s", car2.model);
printf("Rental Rate per Day: ");
scanf("%f", &car2.rentalRatePerDay);
// Input details for the third car
printf("\nInput details for Car 3:\n");
printf("Car ID: ");
scanf("%d", &car3.carID);
printf("Model: ");
scanf("%s", car3.model);
printf("Rental Rate per Day: ");
scanf("%f", &car3.rentalRatePerDay);
// Input the number of rental days
printf("\nEnter the number of rental days: ");
scanf("%d", &rentalDays);
// Calculate and display the total rental cost for each car
printf("\nTotal Rental Cost for Car 1: %.2f\n", calculateRentalCost(car1, rentalDays));
printf("Total Rental Cost for Car 2: %.2f\n", calculateRentalCost(car2, rentalDays));
printf("Total Rental Cost for Car 3: %.2f\n", calculateRentalCost(car3, rentalDays));
return 0;
}
Output:
Input details for Car 1: Car ID: 123 Model: AB2012 Rental Rate per Day: 200 Input details for Car 2: Car ID: 134 Model: XY2013 Rental Rate per Day: 150 Input details for Car 3: Car ID: 135 Model: JU2012 Rental Rate per Day: 180 Enter the number of rental days: 10 Total Rental Cost for Car 1: 2000.00 Total Rental Cost for Car 2: 1500.00 Total Rental Cost for Car 3: 1800.00
Explanation:
In the exercise above,
- The "Car" structure is defined with members 'carID', 'model', and 'rentalRatePerDay'.
- The "calculateRentalCost()" function calculates the total rental cost for a specified number of days for a given car.
- The program prompts the user to input details for three cars and the number of rental days.
- It then calculates and displays the total rental cost for each car.
Flowchart:
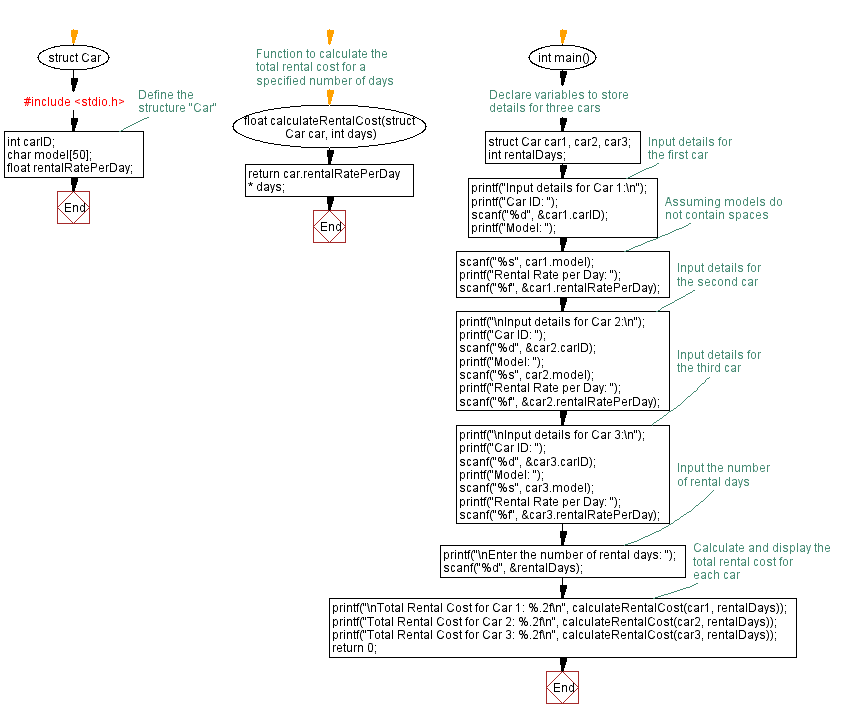
For more Practice: Solve these Related Problems:
- Write a C program to input details for three cars and then display the car with the lowest rental rate per day.
- Write a C program to compute the total rental cost for each car for a given number of days and display the most expensive option.
- Write a C program to store car details in an array of structures, sort the cars based on rental rate in ascending order, and display the sorted list.
- Write a C program to input car details and then search for a car by model name, displaying its details if found or an error message if not.
C Programming Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.