C Program: Print first 10 Fibonacci numbers - While loop
10. Fibonacci Sequence Generation Using a While Loop
Write a C program to find and print the first 10 Fibonacci numbers using a while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int count = 10; // Number of Fibonacci numbers to generate
int firstTerm = 0, secondTerm = 1; // Initialize the first two terms of the sequence
printf("First %d Fibonacci numbers:\n", count);
// Start a while loop to generate Fibonacci numbers
while (count > 0) {
printf("%d, ", firstTerm); // Print the current Fibonacci number
int nextTerm = firstTerm + secondTerm; // Calculate the next Fibonacci number
firstTerm = secondTerm; // Update the first term with the value of the second term
secondTerm = nextTerm; // Update the second term with the calculated next term
count--; // Decrease the count for the next iteration
}
return 0; // Indicate successful program execution
}
Sample Output:
First 10 Fibonacci numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Explanation:
Here are key parts of the above code step by step:
- Variable initialization:
- count: Number of Fibonacci numbers to generate (set to 10).
- firstTerm and secondTerm: Initialize the first two terms of the Fibonacci sequence.
- Print Statement:
- Prints a message indicating the intent to display the first 10 Fibonacci numbers.
- While Loop (Fibonacci Generation):
- Enters a while loop that continues until 'count' becomes 0.
- Prints the current Fibonacci number ('firstTerm').
- Calculates the next Fibonacci number ('nextTerm') by adding the first and second terms.
- Updates the first and second terms for the next iteration.
- Decreases the count for the next iteration.
- Return Statement:
- Indicates successful program execution.
Flowchart:
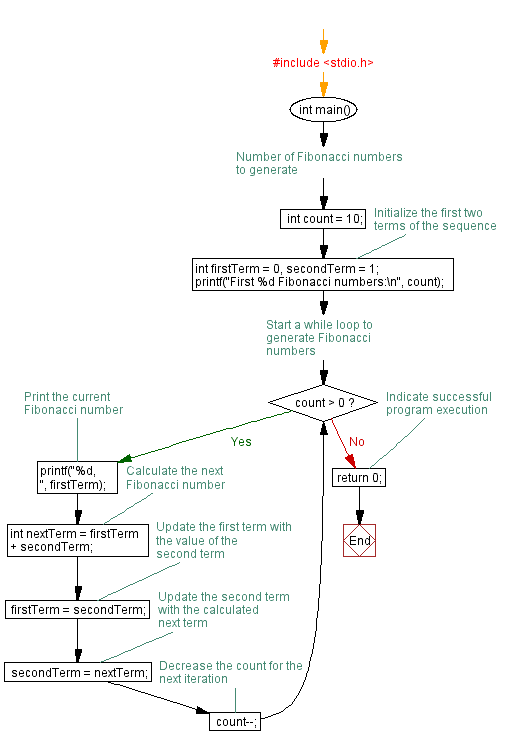
For more Practice: Solve these Related Problems:
- Write a C program to print the first 10 Fibonacci numbers using a while loop and then calculate their sum.
- Write a C program to generate the first 10 Fibonacci numbers using a while loop and display them in reverse order.
- Write a C program to print the first 10 Fibonacci numbers using a while loop and then output the ratio of consecutive Fibonacci numbers.
- Write a C program to generate the first 10 Fibonacci numbers using a while loop and check which of them are prime numbers.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Palindrome check using While loop.
Next: C Program to Print multiplication table using while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.