C++ Exercises: Check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other
Check for Two Adjacent Even or Odd Numbers in Array
Write a C++ program to check a given array of integers. The program will return true if the given array contains either 2 even or 2 odd values all next to each other.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that checks if there are two consecutive even or odd numbers in the array
static bool test(int numbers[], int arr_length)
{
int tot_odd = 0, tot_even = 0; // Initializing counters for odd and even numbers
// Loop through the array to find consecutive even or odd numbers
for (int i = 0; i < arr_length; i++)
{
// Check conditions for consecutive even or odd numbers
if (tot_odd < 2 && tot_even < 2)
{
if (numbers[i] % 2 == 0)
{
tot_even++; // Increment count for even numbers
tot_odd = 0; // Reset count for odd numbers
}
else
{
tot_odd++; // Increment count for odd numbers
tot_even = 0; // Reset count for even numbers
}
}
}
// Return true if there are two consecutive odd or even numbers, otherwise false
return tot_odd == 2 || tot_even == 2;
}
int main()
{
// Different test cases with arrays of integers
int nums1[] = {3, 5, 1, 3, 7}; // No two consecutive even or odd numbers
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 2, 3, 4}; // No two consecutive even or odd numbers
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 3, 5, 5, 5, 5}; // Two consecutive odd numbers
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
int nums4[] = {2, 4, 5, 6}; // Two consecutive even numbers
arr_length = sizeof(nums4) / sizeof(nums4[0]);
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1 1
Visual Presentation:
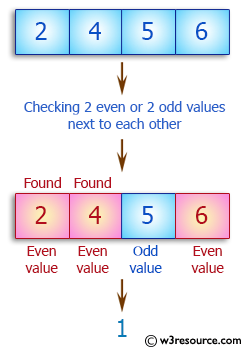
Flowchart:
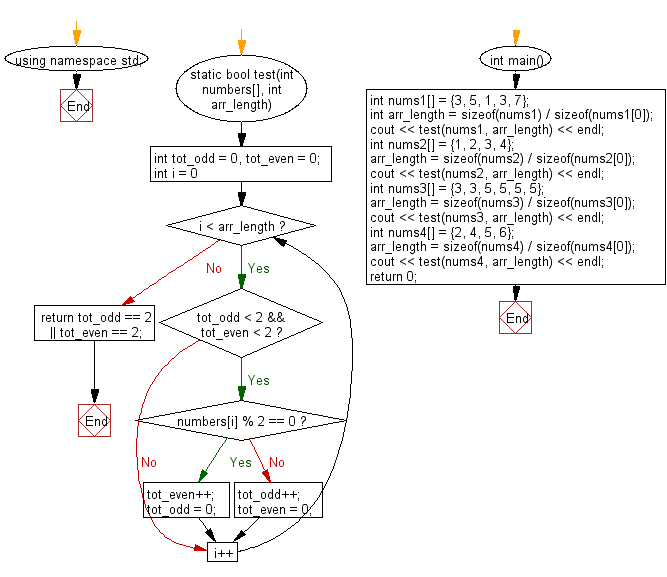
For more Practice: Solve these Related Problems:
- Write a C++ program to check if an array contains at least one instance of two consecutive even numbers or two consecutive odd numbers, and return true if found.
- Write a C++ program that reads an array of integers and outputs true if there are any two adjacent elements that are both even or both odd.
- Write a C++ program to determine if an integer array has any adjacent pair where both numbers are even or both are odd, printing the boolean result.
- Write a C++ program that examines an array and returns 1 if it finds a pair of consecutive even or odd numbers, else 0.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
Next: Write a C++ program to check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other.
What is the difficulty level of this exercise?