C++ Exercises: Count the string 'aa' in a given string and assume 'aaa' contains two 'aa'
Count 'aa' in String
Write a C++ program to count the string "aa" in a given string and assume "aaa" contains two "aa".
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count occurrences of "aa" pairs in the input string 's'
int test(string s)
{
int ctr_aa = 0; // Counter to store the occurrences of "aa" pairs
// Iterate through the string 's' to check for "aa" pairs
for (int i = 0; i < s.length() - 1; i++)
{
// Check if the substring of length 2 starting at index 'i' is equal to "aa"
if (s.substr(i, 2) == "aa")
{
ctr_aa++; // Increment the counter if "aa" is found
}
}
return ctr_aa; // Return the count of "aa" pairs in the string 's'
}
// Main function
int main()
{
// Output the counts of "aa" pairs in different input strings
cout << test("bbaaccaag") << endl;
cout << test("jjkiaaasew") << endl;
cout << test("JSaaakoiaa") << endl;
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
2 2 3
Visual Presentation:
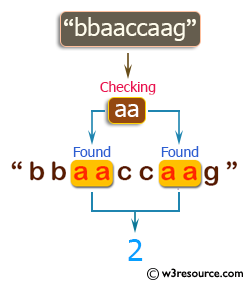
Flowchart:
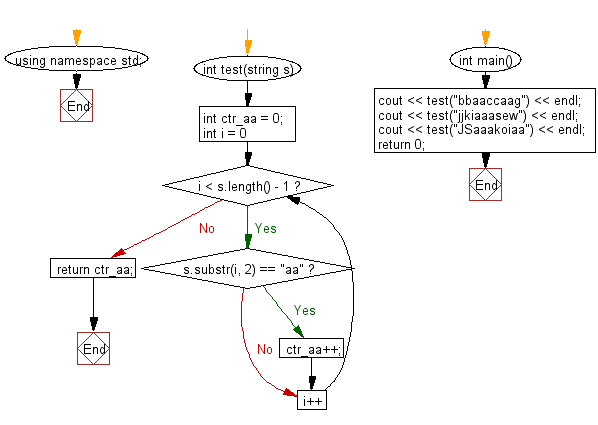
For more Practice: Solve these Related Problems:
- Write a C++ program to count how many times the substring "aa" appears in a string, treating overlapping occurrences correctly.
- Write a C++ program that reads a string and counts occurrences of "aa", considering that "aaa" should be counted as containing two "aa" substrings.
- Write a C++ program to search for the pattern "aa" in an input string and output the total number of occurrences, including overlapping cases.
- Write a C++ program that uses a loop to iterate through a string and count the instances where "aa" appears, accounting for overlap.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string which is n (non-negative integer) copies of the the first 3 characters of a given string. If the length of the given string is less than 3 then return n copies of the string.
Next: Write a C++ program to check if the first appearance of "a" in a given string is immediately followed by another "a".
What is the difficulty level of this exercise?