C++ File handling: Find and replace specific word in text file
C++ File handling: Exercise-6 with Solution
Write a C++ program to find and replace a specific word in a text file.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
// Function to display the content of a file
void displayFileContent(const std::string & filename) {
std::ifstream file(filename); // Open file with given filename
std::string line; // Declare a string to store each line of text
if (file.is_open()) { // Check if the file was successfully opened
std::cout << "File content:" << std::endl; // Displaying a message indicating file content
while (std::getline(file, line)) { // Read each line from the file
std::cout << line << std::endl; // Display each line of the file
}
file.close(); // Close the file
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
}
int main() {
std::ifstream inputFile("test.txt"); // Open the input file named "test.txt" for reading
std::ofstream outputFile("new_test.txt"); // Create or overwrite the output file named "new_test.txt" for writing
if (inputFile.is_open() && outputFile.is_open()) { // Check if both input and output files were successfully opened
std::string line; // Declare a string variable to store each line of text
std::string searchWord = "C++"; // Define the word to search for
std::string replaceWord = "CPP"; // Define the word to replace with
std::cout << "Search word:" << searchWord << std::endl; // Display the word to search for
std::cout << "Replace word:" << replaceWord << std::endl; // Display the word to replace with
std::cout << "\nBefore find and replace:" << std::endl; // Display a message before find and replace
displayFileContent("test.txt"); // Display the content of the input file before find and replace
while (std::getline(inputFile, line)) { // Loop through each line in the input file
size_t pos = line.find(searchWord); // Find the position of the search word in the line
while (pos != std::string::npos) { // Repeat until all occurrences are replaced
line.replace(pos, searchWord.length(), replaceWord); // Replace the search word with the replace word
pos = line.find(searchWord, pos + replaceWord.length()); // Find the next occurrence of the search word
}
outputFile << line << "\n"; // Write the modified line to the output file
}
inputFile.close(); // Close the input file
outputFile.close(); // Close the output file
std::cout << "After find and replace:" << std::endl; // Display a message after find and replace
displayFileContent("new_test.txt"); // Display the content of the output file after find and replace
std::cout << "\nWord replaced successfully." << std::endl; // Display a success message
} else {
std::cout << "\nFailed to open the files." << std::endl; // Display an error message if file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Search word:C++ Replace word:CPP Before find and replace: File content: C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. After find and replace: File content: CPP is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern CPP currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide CPP compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. Word replaced successfully.
Explanation:
In the above exercise -
- Defines a function displayFileContent that takes a filename as an argument. This function opens the specified file, reads its content line by line, and displays each line on the console.
- Inside the main() function, it opens an input file named "test.txt" using std::ifstream and an output file named "new_test.txt" using std::ofstream.
- It checks if both files were successfully opened using the is_open function.
- It calls the displayFileContent function to display the input file content before the find and replace operation.
- It enters a while loop to read each line from the input file using std::getline.
- Inside the loop, it uses the find function to search for the searchWord within the line. If it finds a match, it enters another loop to replace all occurrences of the searchWord with the replaceWord using the replace function.
- After modifying the line, it writes the modified line to the output file using the output stream operator <<.
- After the loop ends, it closes both the input and output files.
- It calls the displayFileContent function again to display the output file content after the find and replace operation.
- Finally, it prints a message indicating that the word was replaced successfully.
Flowchart:
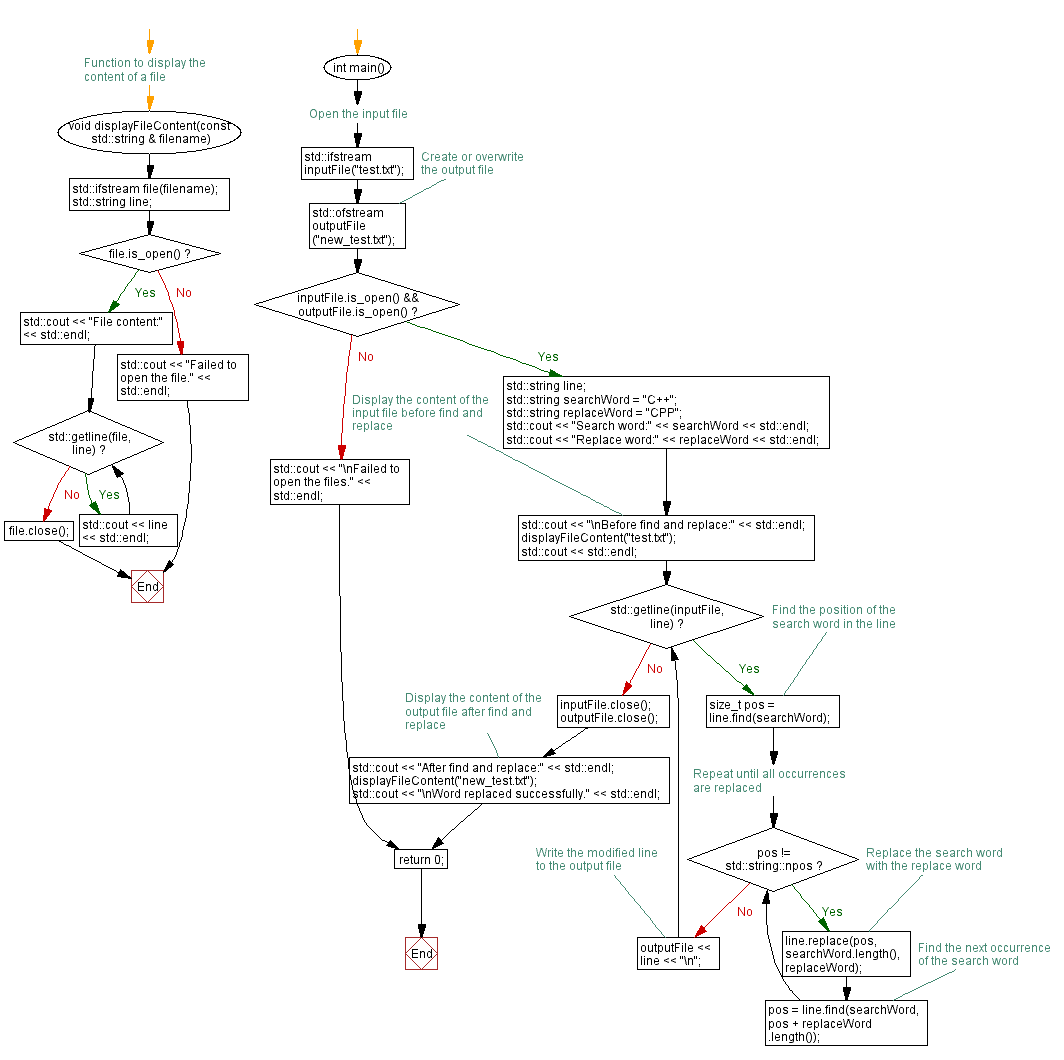
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Copy contents from one text file to another.
Next C++ Exercise: Append data to existing text file.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/file-handling/cpp-file-handling-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics