C++ Exercises: Check whether a given number is a power of two or not
1. Check if Number is a Power of Two
Write a C++ program to check whether a given number is a power of two or not.
Visualization of powers of two from 1 to 1024:
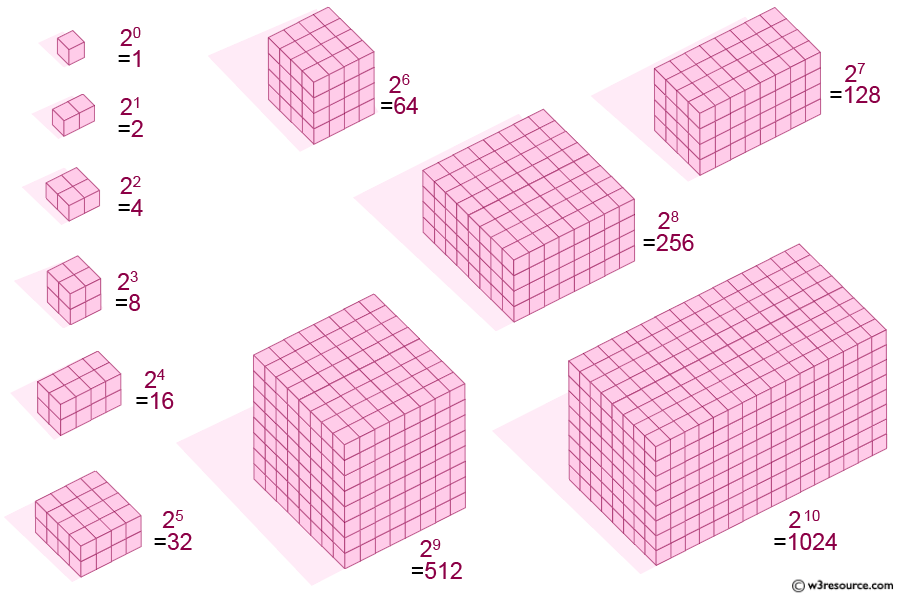
Sample Solution:
C++ Code :
#include <iostream> // Include input/output stream library
#include <cmath> // Include math functions
using namespace std; // Using standard namespace
// Function to check if a number is a power of two
string Powers_of_Two(int n) {
// Loop through integers from 0 to maximum integer value
for (int x = 0; x < INT_MAX; x++)
{
// Check if 2 raised to the power of 'x' is equal to 'n'
if (pow(2, x) == n)
{
return "True"; // Return "True" if 'n' is a power of 2
}
// If 2 raised to the power of 'x' exceeds 'n', break the loop
else if (pow(2, x) > n)
{
break;
}
}
return "False"; // Return "False" if 'n' is not a power of 2
}
int main() {
// Test cases
cout << "Is 8 a power of 2: " << Powers_of_Two(8) << endl;
cout << "Is 256 a power of 2: " << Powers_of_Two(256) << endl;
cout << "Is 124 a power of 2: " << Powers_of_Two(124) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Is 8 is power of 2: True Is 256 is power of 2: True Is 124 is power of 2: False
Flowchart:
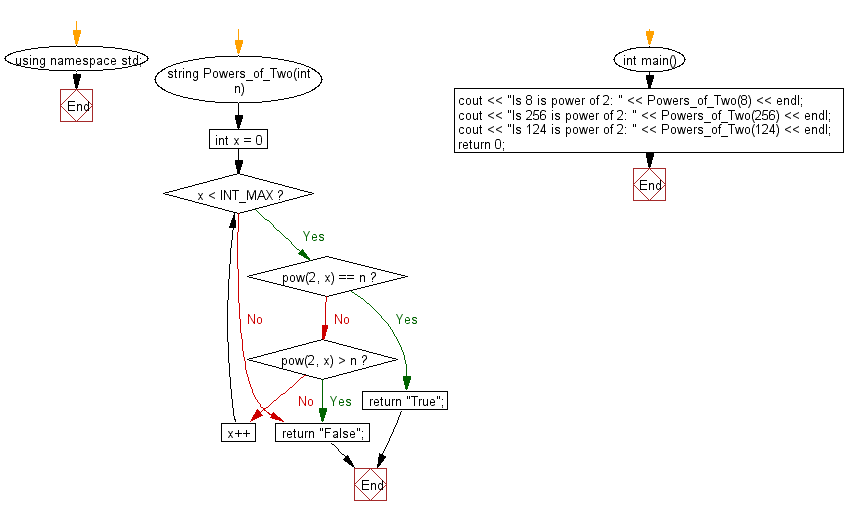
For more Practice: Solve these Related Problems:
- Write a C++ program that determines if a given integer is a power of two using bit manipulation (n & (n-1) technique).
- Write a C++ program to check if a number is a power of two by recursively dividing the number by 2 until it reaches 1.
- Write a C++ program that reads an integer and uses a loop to continuously divide by 2, verifying if the final result is 1.
- Write a C++ program to implement a function that returns true if an input number is a power of two, using logarithms and comparing with an integer value.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: C++ Math Exercises Home.
Next: Write a C++ program to check the additive persistence of a given number.
What is the difficulty level of this exercise?