C++ Recursion: Checking palindrome strings using recursive function
C++ Recursion: Exercise-8 with Solution
Write a C++ program to implement a recursive function to check if a given string is a palindrome.
A palindrome is a word, number, phrase, or other sequence of symbols that reads the same backwards as forwards, such as madam or racecar, the date and time 12/21/33 12:21, and the sentence: "A man, a plan, a canal – Panama".
Sample Solution:
C Code:
// Recursive function to check if a string is a palindrome
#include <iostream> // Including the Input/Output Stream Library
#include <string> // Including the String Library
#include <algorithm> // Including the Algorithm Library
// Recursive function to check if a string is a palindrome
bool isPalindrome(const std::string & text, int start, int end) {
// Base case: when start >= end, the string is a palindrome
if (start >= end)
return true;
// Recursive case: check if the characters at start and end indices are equal,
// and recursively check the remaining characters
if (text[start] != text[end])
return false;
return isPalindrome(text, start + 1, end - 1);
}
int main() {
std::string text; // Declaring a variable to store the input string
std::cout << "Input a string: ";
std::getline(std::cin, text); // Taking input for the string
// Remove any spaces from the input string
text.erase(std::remove(text.begin(), text.end(), ' '), text.end());
// Check if the string is a palindrome using recursion
bool palindrome = isPalindrome(text, 0, text.length() - 1); // Checking if the input string is a palindrome
// Display the result
if (palindrome)
std::cout << "The string is a palindrome." << std::endl;
else
std::cout << "The string is not a palindrome." << std::endl;
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Input a string: madam The string is a palindrome.
Input a string: abc The string is not a palindrome.
Flowchart:
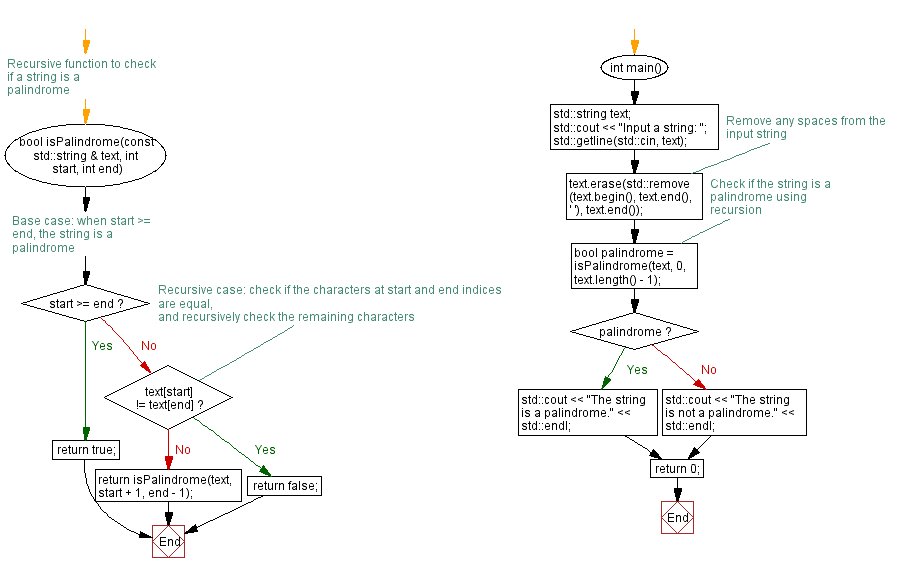
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Calculating the power of a number using recursive function.
Next C++ Exercise: Reversing a linked list with recursive function.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics