C++ Stack Exercises: Reverse a stack (using a dynamic array) elements
22. Reverse the Elements of a Dynamic Array-Based Stack
Write a C++ program that reverses the stack (using a dynamic array) elements.
Test Data:
Input the size of the stack: 8
Input some elements onto the stack:
Stack elements are: 4 7 5 3 1
Reverse the said stack elements:
Stack elements are: 1 3 5 7 4
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
// Class representing a Stack
class Stack {
public:
int top; // Index of top element
int capacity; // Maximum size of the stack
int* arra; // Pointer to the array storing elements
// Constructor to initialize the stack with a given size
Stack(int size) {
arra = new int[size]; // Allocate memory for the stack array
top = -1; // Initialize top index to -1 (empty stack)
capacity = size; // Set the maximum capacity of the stack
}
// Function to push an element onto the stack
bool push(int x) {
if (isFull()) {
cout << "Stack is full" << endl; // Display overflow message if the stack is full
return false;
}
// Add element to array by incrementing top index
arra[++top] = x;
return true;
}
// Function to pop an element from the stack
int pop() {
if (isEmpty()) {
cout << "Stack underflow" << endl; // Display underflow message if the stack is empty
return 0;
}
// Return the top element and decrement the index of the top element
return arra[top--];
}
// Function to peek the top element of the stack without removing it
int peek() {
if (isEmpty()) {
cout << "Stack is empty" << endl; // Display message if the stack is empty
return 0;
}
// Return the top element without modifying the top index
return arra[top];
}
// Function to check if the stack is empty
bool isEmpty() {
// Stack is empty if top index is -1
return (top < 0);
}
// Function to check if the stack is full
bool isFull() {
// Stack is full if top index is equal to capacity - 1
return (top >= capacity - 1);
}
// Function to display the elements of the stack
void display() {
if (top < 0) {
cout << "Stack is empty" << endl; // Display message if the stack is empty
return;
}
cout << "\nStack elements are: ";
for (int i = top; i >= 0; i--)
cout << arra[i] << " "; // Display the elements of the stack
cout << endl;
}
};
// Function to sort the elements of a stack in descending order
void sort_stack(int* stackArray, int& top) {
int temp;
for (int i = 0; i <= top; i++) {
for (int j = i + 1; j <= top; j++) {
if (stackArray[j] > stackArray[i]) {
// Swap elements to arrange them in descending order
temp = stackArray[i];
stackArray[i] = stackArray[j];
stackArray[j] = temp;
}
}
}
}
int main() {
int size;
cout << "Input the size of the stack: ";
cin >> size; // Take input for the size of the stack
Stack stk(size); // Create a stack of the given size
cout << "\nInput some elements onto the stack:";
stk.push(1);
stk.push(3);
stk.push(5);
stk.push(7);
stk.push(4);
stk.display(); // Display the elements of the stack
cout << "\nAfter sorting the said stack elements:";
sort_stack(stk.arra, stk.top); // Sort the stack elements in descending order
stk.display(); // Display the sorted elements of the stack
return 0;
}
Sample Output:
Input the size of the stack: 8 Input some elements onto the stack: Stack elements are: 4 7 5 3 1 Reverse the said stack elements: Stack elements are: 1 3 5 7 4 Input 2 more elements onto the said stack: Stack elements are: 40 70 1 3 5 7 4 Reverse the said stack elements: Stack elements are: 4 7 5 3 1 70 40
Flowchart:
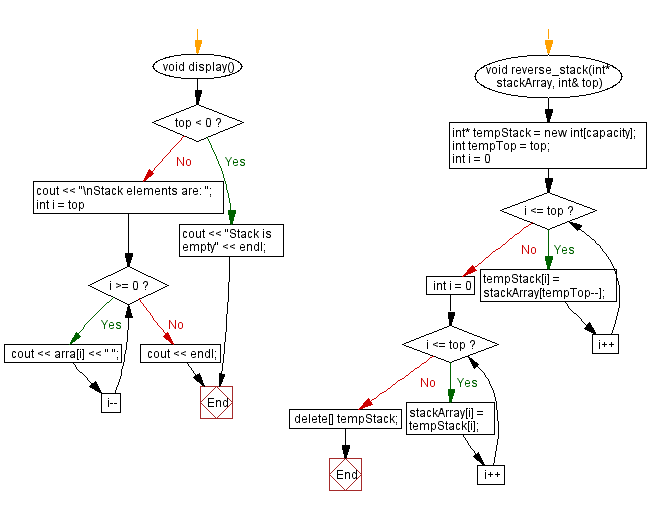
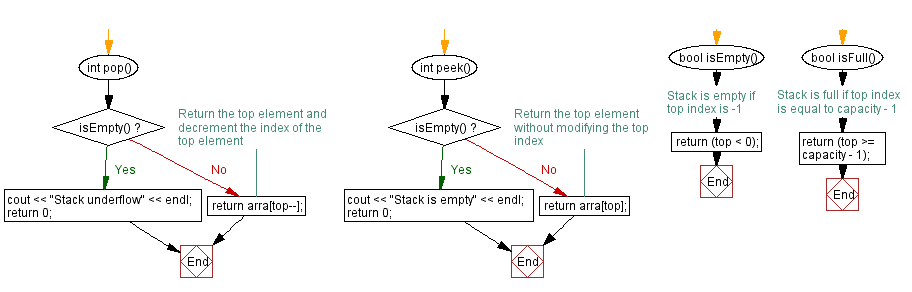
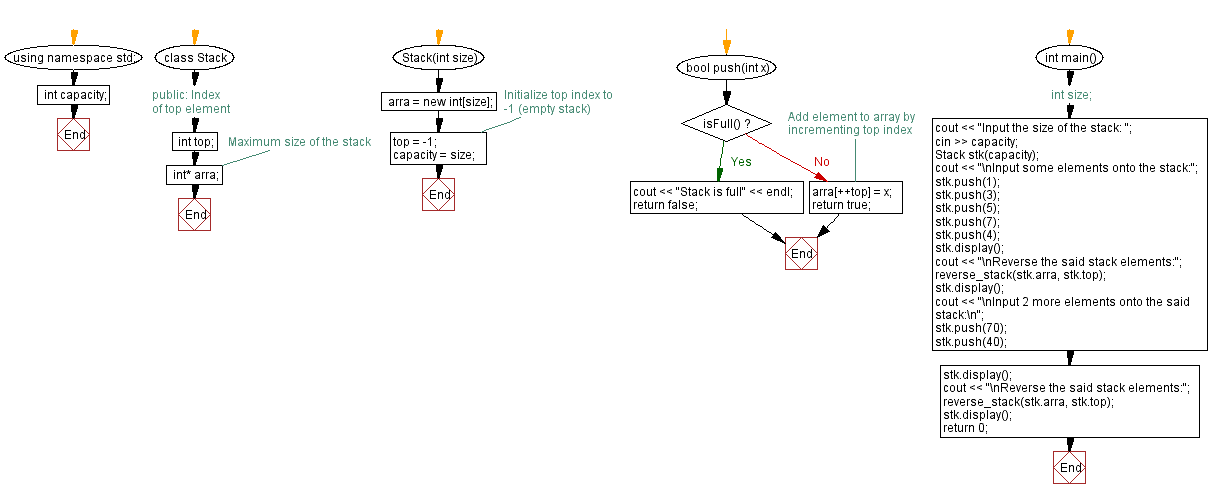
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse a stack implemented with a dynamic array and print the reversed sequence.
- Develop a C++ program that dynamically creates a stack and reverses its elements using in-place swapping.
- Design a C++ program to implement a dynamic array-based stack and then display its reverse without additional memory allocation.
- Implement a C++ program to build a dynamic stack and reverse its order by iterating from the last element to the first.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Sort a stack (using a dynamic array) elements.
Next C++ Exercise: Middle element of a stack (using a dynamic array).
What is the difficulty level of this exercise?