C++ Stack Exercises: Implement a stack using a vector with push, pop operations
24. Implement Stack using Vector with Push, Pop, and Top Check
Write a C++ program to implement a stack using a vector with push and pop operations. Check if the stack is empty or not and find the top element of the stack.
Test Data:
Create a stack object:
Is the stack empty? Yes
Input and store (using vector) some elements onto the stack:
Stack elements are: 1 2 3 4 5
Top element is 5
Sample Solution:
C++ Code:
#include <iostream>
#include <vector>
using namespace std;
// Class representing a Stack using a vector
class Stack {
private:
vector<int> elements; // Vector to store elements
public:
// Function to push an element onto the stack
void push(int element) {
// Add element to the end of the vector
elements.push_back(element);
}
// Function to pop an element from the stack
void pop() {
if (elements.empty()) {
cout << "Stack is full" << endl; // Display full message if the stack is empty
} else {
// Remove the last element from the vector
elements.pop_back();
}
}
// Function to get the top element of the stack
int top() {
if (elements.empty()) {
cout << "Stack is empty" << endl; // Display empty message if the stack is empty
return 0;
} else {
// Return the last element in the vector
return elements.back();
}
}
// Function to check if the stack is empty
bool empty() {
// Check if the vector is empty
return elements.empty();
}
// Function to display the elements of the stack
void display() {
vector<int> v = elements;
if (v.empty()) {
cout << "Stack is empty" << endl; // Display empty message if the stack is empty
return;
}
cout << "Stack elements are: ";
for (int i = 0; i < v.size(); i++) {
cout << v[i] << " "; // Display the elements of the stack
}
cout << endl;
}
};
int main() {
Stack stack; // Create a stack object
cout << "Create a stack object:\n";
cout << "Is the stack empty? " << (stack.empty() ? "Yes" : "No") << endl;
cout << "\nInput and store (using vector) some elements onto the stack:\n";
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
stack.display();
cout << "\nTop element is " << stack.top() << endl;
cout << "\nRemove two elements from the said stack:\n";
stack.pop();
stack.pop();
stack.display();
cout << "\nTop element is " << stack.top() << endl;
return 0;
}
Sample Output:
Create a stack object: Is the stack empty? Yes Input and store (using vector) some elements onto the stack: Stack elements are: 1 2 3 4 5 Top element is 5 Remove two elements from the said stack: Stack elements are: 1 2 3 Top element is 3
Flowchart:
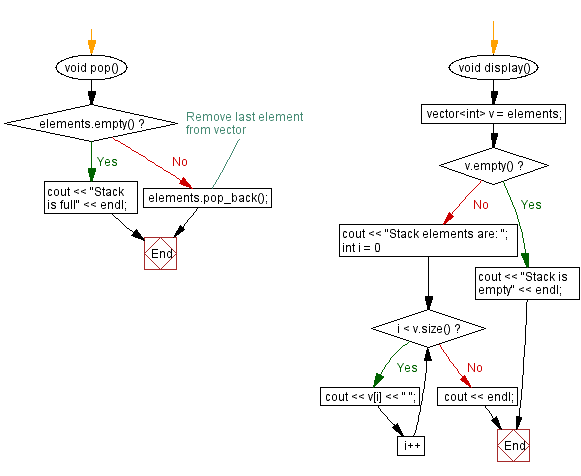
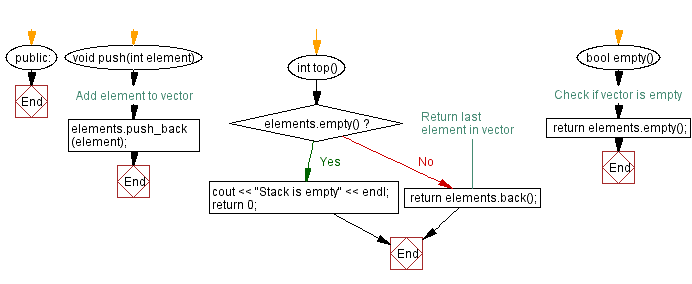
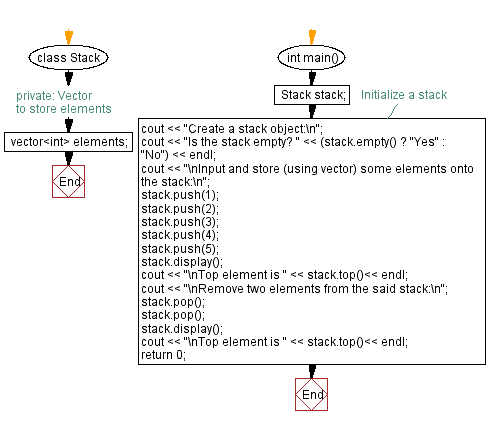
For more Practice: Solve these Related Problems:
- Write a C++ program to simulate stack operations using a vector and check for emptiness before pop operations.
- Develop a C++ program that creates a vector-based stack and displays the top element after each operation.
- Design a C++ program to implement a stack using C++ vectors and verify stack status after each push/pop.
- Implement a C++ program to manage stack operations with a vector, including custom error handling for empty stacks.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Middle element of a stack (using a dynamic array).
Next C++ Exercise: Sort the stack (using a vector) elements.
What is the difficulty level of this exercise?